WordPress TinyMCE: How to Add Styles, Buttons, Fonts, Dropdowns & Popups
Back with the release of WordPress 3.9 the TinyMCE editor was updated to version 4.0 which included some cool new functions. The new TinyMCE looks a look cleaner (really matches the WP dashboard) and it has some really nice added functionality.
A lot of my old themes and plugins had to be updated to work with the new TinyMCE so I spent some time digging through the API and figuring some cool stuff out. Below are some examples showing how to extend the functionality of the TinyMCE.
I’m not going to walk you through all the steps or what the code means exactly (this is intended for developers) but I will provide you with the exact code you can copy/paste in your theme or plugin and then tweak accordingly.
Important: This guide is specifically for the tinyMCE editor used for the WordPress “classic editor” it’s not intended for those using Gutenberg. If you are using Gutenberg on your site you shouldn’t really be messing around with the tinyMCE as that’s a function of the old WP editor.
How to Enable the Font Size & Font Family Select Dropdowns
By default the custom fonts and font sizes are not added into the TinyMCE editor. The function below will add both of these dropdowns to the far left of the editor in the second row. Simply change where it says ‘mce_buttons_2’ if you want it in a different row (ex: use ‘mce_buttons_3’ for the 3rd row).
// Enable font size & font family selects in the editor.
add_filter( 'mce_buttons_2', function( $buttons ) {
array_unshift( $buttons, 'fontselect' );
array_unshift( $buttons, 'fontsizeselect' );
return $buttons;
} );
How to Add Custom Font Sizes
By default the font sizes are set to pt values which isn’t always ideal. I prefer to use pixel values (12px, 13px, 14px, 16px..etc) and to provide more options for grater flexibility. The function below will alter the default font size options in the dropdown selector.
// Customize the classic editor font size dropdown.
add_filter( 'tiny_mce_before_init', function( $init_array ) {
$init_array['fontsize_formats'] = "9px 10px 12px 13px 14px 16px 18px 21px 24px 28px 32px 36px";
return $init_array;
} );
How to Add Custom Font Options
The default font options in the font family selector are all “web-safe” fonts by default, but what if you wanted to add more fonts to the selector? Maybe some Google Fonts? We’ll it’s really easy have a look at the example below.
// Add custom Fonts to the Fonts list.
add_filter( 'tiny_mce_before_init', function( $initArray ) {
$initArray['font_formats'] = 'Lato=Lato;Andale Mono=andale mono,times;Arial=arial,helvetica,sans-serif;Arial Black=arial black,avant garde;Book Antiqua=book antiqua,palatino;Comic Sans MS=comic sans ms,sans-serif;Courier New=courier new,courier;Georgia=georgia,palatino;Helvetica=helvetica;Impact=impact,chicago;Symbol=symbol;Tahoma=tahoma,arial,helvetica,sans-serif;Terminal=terminal,monaco;Times New Roman=times new roman,times;Trebuchet MS=trebuchet ms,geneva;Verdana=verdana,geneva;Webdings=webdings;Wingdings=wingdings,zapf dingbats';
return $initArray;
} );
Notice how I added “Lato” to the list in the code above? It’s that simple! In my Total WordPress theme I actually loop through any custom font’s used on the site as defined in the theme panel and add them to the select box so they are also available while editing your posts/pages (sweet).
But the code above only adds the font-family to the drop-down it won’t magically load the script so that when you change your text in the editor you can actually see that custom font applied to it…That’s what the code below does!
// Add Google Scripts for use with the editor.
add_action( 'admin_init', function() {
$font_url = 'http://fonts.googleapis.com/css?family=Lato:300,400,700';
add_editor_style( str_replace( ',', '%2C', $font_url ) );
} );
The code above loads the custom font regardless if the font is actually being used. I only recommend using code like this if you are loading a few fonts as to not slow things down.
Editor Formats (styles)
Remember the “Styles” dropdown in WP 3.8? That was pretty cool! You could use it to add custom classes for use with the post editor so you could apply custom styles to different elements. Well, in WordPress 3.9 you can still add styles, however, it has been renamed to “Formats” so it works differently. Below is an example of how to enable the Formats dropdown and also add some new items to it.
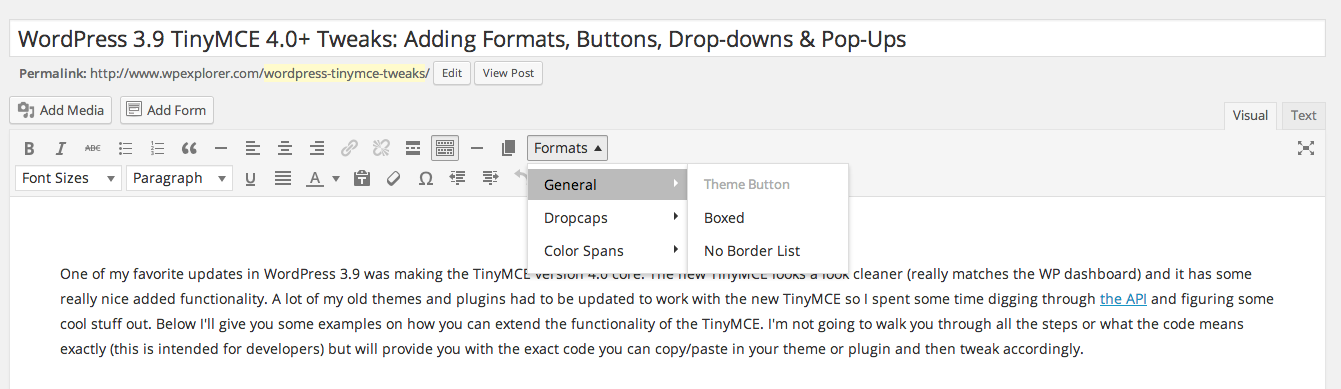
How to Enable the Formats Dropdown Menu
This is actually done in the same manner prior to WP 3.9 but I’m sharing in case you didn’t know how to do it.
// Enable the formats dropdown in the tiny mce editor.
add_filter( 'mce_buttons', function( $buttons ) {
array_push( $buttons, 'styleselect' );
return $buttons;
} );
How to Add New Items to the Formats Dropdown
Adding new items is super easy. Below is a snippet showing how to add a theme button and highlight option.
// Add new styles to the TinyMCE "formats" menu dropdown.
add_filter( 'tiny_mce_before_init', function( $settings ) {
$new_styles = [
arra[
'title' => __( 'Custom Styles', 'text_domain' ),
'items' => [
[
'title' => __( 'Theme Button', 'text_domain' ),
'selector' => 'a',
'classes' => 'button'
],
[
'title' => __( 'Highlight', 'text_domain' ),
'inline' => 'span',
'classes' => 'highlight',
],
],
],
];
$settings['style_formats_merge'] = true;
$settings['style_formats'] = json_encode( $new_styles );
return $settings;
} );
Please notice how I’ve added “$settings['style_formats_merge'] = true;
” to the code above. This extra bit of code makes sure your options are added to the formats dropdown menu along with any other options previously registered by your theme or 3rd party plugins.
How to Add a Simple Custom MCE Button
Adding a new button to the TinyMCE editor is especially useful for shortcodes. As a user it is a real pain to remember shortcodes, so having a button to easily insert one is very convenient.
I’m not saying to add 100’s of buttons to the TinyMCE for all your shortcodes. I hate when developers do this, it’s such bad practice and looks horrible. But if you add one or a few I’ll let it pass π
If you do want to add a bunch of buttons for inserting stuff into the editor, then you should create a submenu as I will explain in the next section of this tutorial.
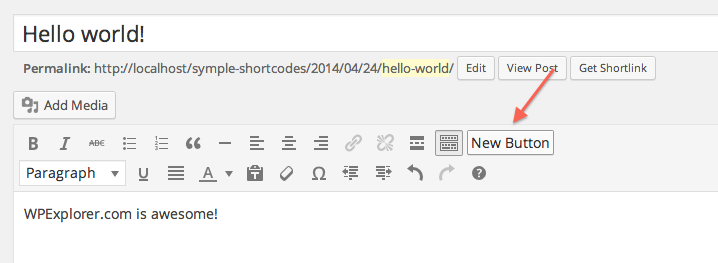
PHP Code – Declare your New MCE Plugin
The following code will declare your new MCE plugin so that it shows up in the editor.
// Add action hooks for registering a custom mce button.
add_action('admin_head', function() {
// check user permissions
if ( ! current_user_can( 'edit_posts' ) && ! current_user_can( 'edit_pages' ) ) {
return;
}
// check if WYSIWYG is enabled
if ( 'true' == get_user_option( 'rich_editing' ) ) {
add_filter( 'mce_external_plugins', 'myprefix_add_tinymce_plugin' );
add_filter( 'mce_buttons', 'myprefix_register_mce_button' );
}
} );
// Declare your custom mce-button script.
function myprefix_add_tinymce_plugin( $plugin_array ) {
$plugin_array['my_mce_button'] = get_template_directory_uri() . '/js/mce-button.js';
return $plugin_array;
}
// Register new button in the editor
function myprefix_register_mce_button( $buttons ) {
array_push( $buttons, 'my_mce_button' );
return $buttons;
}
Javascript Code – Adds the Button to the Editor
This code below should go in the js file registered in the snippet above in the “myprefix_add_tinymce_plugin” function. This will add a new text button that says “New Button” in the editor and when clicked it will insert the text “WPExplorer.com is awesome!”.
(function() {
tinymce.PluginManager.add( 'my_mce_button', function( editor, url ) {
editor.addButton('my_mce_button', {
text: 'New Button',
icon: false,
onclick: function() {
editor.insertContent('WPExplorer.com is awesome!');
}
});
});
})();
How to Add a Custom Icon to Your New MCE Button
Above I showed you how to add a new button that will display as “New Button” in the editor, the following will show you how to add a custom icon to your button so that it stands out.
Load a stylesheet with your CSS
Use this function to load a new stylesheet in the WordPress admin where you can add custom CSS to target things such as your custom editor buttons.
// Load a custom CSS file in the wp backend.
add_action( 'after_setup_theme', function() {
add_editor_style( trailingslashit( get_template_directory_uri() ) . 'css/editor-style.css' );
} );
Button Icon CSS
This is the CSS to add the the stylesheet loaded previously.
i.my-mce-icon {
background-image: url('YOUR ICON URL');
}
Tweak your Javascript
Now simply tweak the javascript which you added previously to remove the text parameter and modify the icon parameter which was previously set to “false” so that it has a custom classname.
(function() {
tinymce.PluginManager.add('my_mce_button', function( editor, url ) {
editor.addButton( 'my_mce_button', {
icon: 'my-mce-icon',
onclick: function() {
editor.insertContent('WPExplorer.com is awesome!');
}
});
});
})();
How to Add a Button with a Submenu
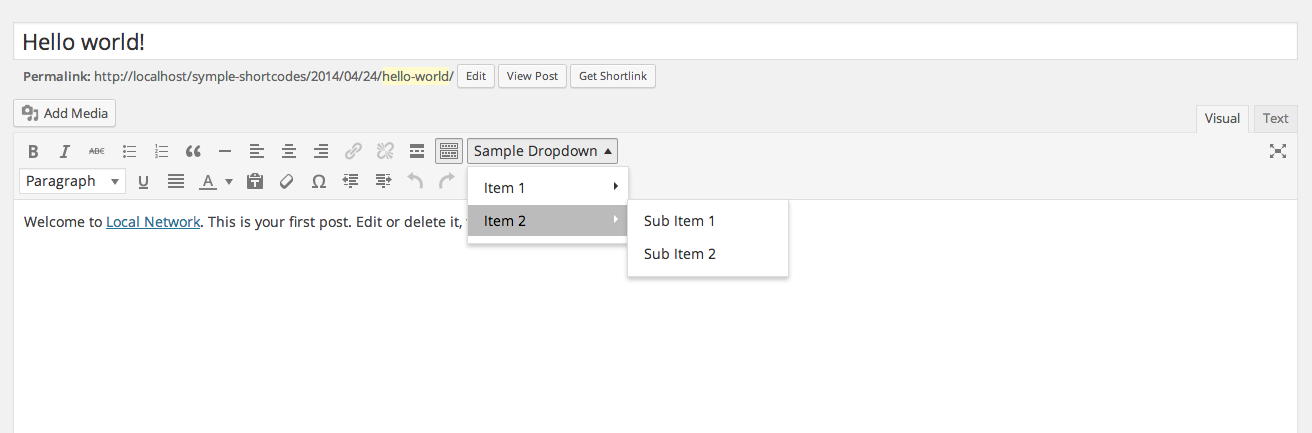
Earlier I mentioned that adding a ton of new icons to the TinyMCE bar is a bad idea (and it is). The code below is an example of how you can edit your javascript to display a submenu with multiple options when clicking on the button.
(function() {
tinymce.PluginManager.add('my_mce_button', function( editor, url ) {
editor.addButton( 'my_mce_button', {
text: 'Sample Dropdown',
icon: false,
type: 'menubutton',
menu: [
{
text: 'Item 1',
menu: [
{
text: 'Sub Item 1',
onclick: function() {
editor.insertContent('WPExplorer.com is awesome!');
}
},
{
text: 'Sub Item 2',
onclick: function() {
editor.insertContent('WPExplorer.com is awesome!');
}
}
]
},
{
text: 'Item 2',
menu: [
{
text: 'Sub Item 1',
onclick: function() {
editor.insertContent('WPExplorer.com is awesome!');
}
},
{
text: 'Sub Item 2',
onclick: function() {
editor.insertContent('WPExplorer.com is awesome!');
}
}
]
}
]
});
});
})();
How to Add a Settings Popup Window to Insert a Shortcode
In the example above you may have noticed that every button simply inserts the text “WPExplorer.com is awesome!” which is cool, but perhaps you want display a field where you can enter the custom text that will be inserted.
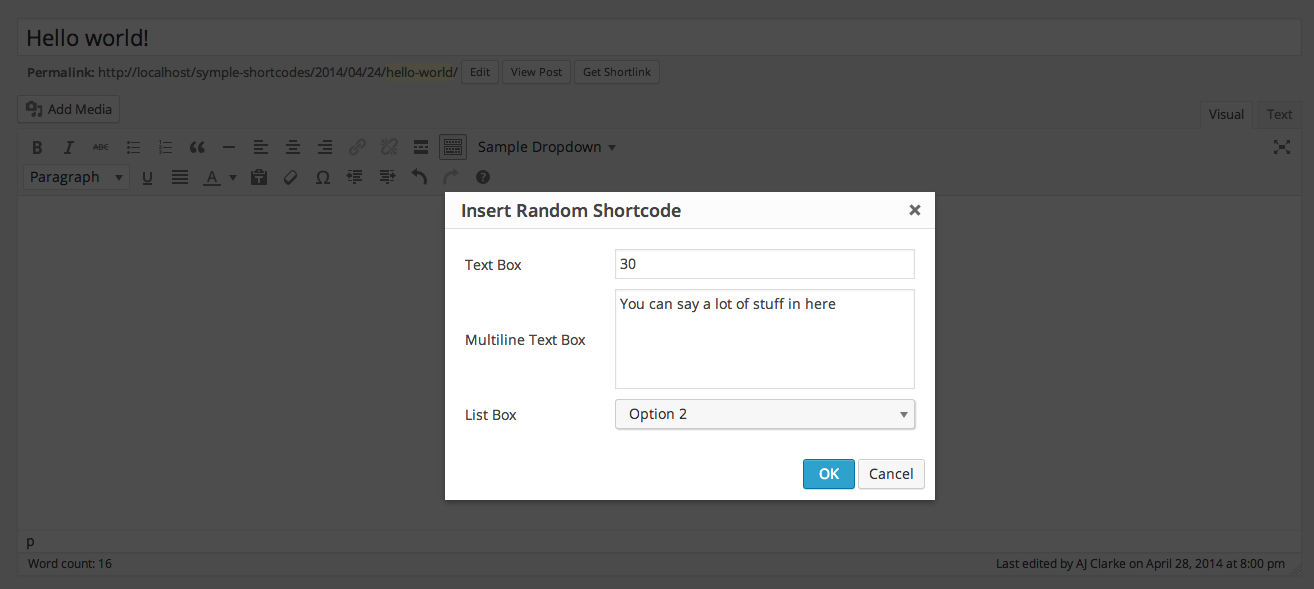
The following snippet shows how to create a popup window with multiple settings that will insert a shortcode based on user input.
(function() {
tinymce.PluginManager.add('my_mce_button', function( editor, url ) {
editor.addButton( 'my_mce_button', {
text: 'Sample Dropdown',
icon: false,
type: 'menubutton',
menu: [
{
text: 'Item 1',
menu: [
{
text: 'Pop-Up',
onclick: function() {
editor.windowManager.open( {
title: 'Insert Random Shortcode',
body: [
{
type: 'textbox',
name: 'textboxName',
label: 'Text Box',
value: '30'
},
{
type: 'textbox',
name: 'multilineName',
label: 'Multiline Text Box',
value: 'You can say a lot of stuff in here',
multiline: true,
minWidth: 300,
minHeight: 100
},
{
type: 'listbox',
name: 'listboxName',
label: 'List Box',
'values': [
{text: 'Option 1', value: '1'},
{text: 'Option 2', value: '2'},
{text: 'Option 3', value: '3'}
]
}
],
onsubmit: function( e ) {
editor.insertContent( '[random_shortcode textbox="' + e.data.textboxName + '" multiline="' + e.data.multilineName + '" listbox="' + e.data.listboxName + '"]');
}
});
}
}
]
}
]
});
});
})();
This is fantastic! Thanks for the great breakdown of different methods. Any comment on how these methods will work in older versions of WP/TinyMCE?
I have fallback methods in my own products, but its not something I added to this post because to be honest no one should be using old version of WordPress π
I agree.
Wow, is this an amazing and informative post! Thanks so much for the code hints. I’m going to be using several of these, although i don’t like to give my “editors” too much control. Might not extend the fonts or google fonts. Thanks again!
You are welcome π For a blog I wouldn’t do that either, but for a business site having fonts available might be useful for creating some compelling landing pages. Good luck!
great post, like your self i did sone digging and worked these out while wp 3.9 was in beta, i hate theme devs that dont beta test leaving their clients a frustrating wait for updates especialy with auto update, And please please theme devs put shortcodes in a plugin
What a great collection of snippets! Will definitely get some use out of this. Thanks AJ!
Ditto!
Hi.
Great article!
I wanted to ask if it was possible to add the button “add media” and the advanced editor in textarea field in Pop-Up Window.
Thanks
I’m sure it wouldn’t be very hard to do, but I haven’t dug into it yet. I might check it out next month though as I update another one of my plugins and if I do I’ll update the post. If you find anything out there, let me know!
Media button:
Epic ! Update several free plugins with this piece of code. Any idea to customize span generated by listbox? Find no documentation for this. I want to add a html class to style it.
Depends on what you need to do…If you want something more advanced what you can do is use the “url” parameter (see the API) this way you can control everything 100%
Thanks will look into this ^^
AJ, I realize you intentionally left out the details since you presume all that read this are developers. I created my wife’s author website and have used the TinyMCE Advanced plugin to control font types and sizes. With the upgrade it’s now missing by default (as you point out). Could you please answer one question? Where do I insert the code to bring these features back? When I inserted the code into the TinyMCE Advanced plugin, nothing changed. I suspect this code needs to be entered into my TwentyTen theme. Again, I’m sorry for the low level question. Thanks again for the code!
Hi Chuck, I’ve never used the TinyMCE Advanced plugin so I’m not sure how it’s supposed to work. Have you tried posting the question on the forum, maybe you can still add these via the plugin but the settings might have changed for WP 3.9?
The code above would be paste into your functions.php (preferably in a child theme).
Unlike Chuck I am not a hot shot programmer. Your code is really pumping up my TinyMCE kit. I wonder how difficult it is to, also or alternatively, show CUFON fonts in the pull down list for FONT FAMILY choice.
I have a real need to use a CUFON font in various text blocks on the fly.
You could use wp_localize_script to store an array of the CUFON Fonts for use.
AJ, thanks so much for the rapid response. Actually the solution was rather simple. Instead of going into the plugin editor, which requires a working knowledge of the code, I went to SETTINGS and then clicked on the TinyMCE Advanced plugin, and there was a list of unused drag and drop buttons, two of which were font family and font sizes. But to do the other things you referenced would definitely entail tweaking the funciton.php file. Thanks again for your generosity. I’m way out of my league. π
Hi AJ Clarke,
For Pop-up Window, could you interact with get post or page in dropdown field?
So appreciate and more useful if you can drop this option in this tutorial.
Sorry I’m not sure what you mean.
Yes, your tutorial is very nice helps for me. Otherwise it would be add one more interactive option like “Show posts by category”.
e.g: when we click on shortcode button and show a popup that contain fields select category and number of post. Could you show how to pass category into javascript shortcode? or are there anyways to make it work?
Thanks.
Yes you can do this, but it is a bit too advanced for this post. Would be it’s on post in itself π
Thank you for this awesome reference! 3.9 broke a whole bunch of editor customizations in my blank theme and this saved my butt. You should get a link in the WordPress codex because it’s out of date now!
Glad it helped you our Adam. A link from the CODEX would be nice, you should recommend it π Thanks!
Done!
Have you figured out how to customize the text color palatte? This no longer works: $options[‘theme_advanced_text_colors’] = ‘ffffff,ba192c,20b7dd’;
Sorry Adam I didn’t see your comment earlier! I have not looked into this, have you figured it out yet?
Adam,
I don’t know if you’re still looking for the answer. I was looking into the same thing and found this:
http://urosevic.net/wordpress/tips/custom-colours-tinymce-4-wordpress-39/
Thank you for sharing, however, this is no longer needed because in one of the latest WordPress updates (I think 4.0) they re-introduced the ability to choose custom colors natively.
Thanks for the very helpful post.
I’m not sure I’m a fan of the new styleselect. Anyone else feel this way? The default content repeats items that are already in the toolbar. If I add my custom styles to the menu, the repeated default content will confuse my clients. But I agree with what AJ says – I don’t want to remove the default content because plugins might use it. (Although I hope they don’t.)
So that all kind ruins the easy option for adding custom styles. The only other option seems to be adding a custom button, requiring Javascript, which I am admittedly pretty rusty at.
For now, I am taking the default content off, despite the disadvantages.
As long as your function can be overwritten via a child theme (using function_exists check or a filter) then I don’t see any problem π
You can also remove specific ones exclusively, I didn’t add it in this post but you can probably find out how doing a Google search.
Hi,
I would like the style also applies to items in the dropdown list, how ?
I tried this:
array( ‘title’ => ‘Red Uppercase Text’, ‘inline’ => ‘span’, ‘styles’ => array( ‘color’ => ‘red’, ‘fontWeight’ => ‘bold’, ‘textTransform’ => ‘uppercase’)),
There is that color does not work. :/
Thanks
To be honest I haven’t looked into that yet so I’m not sure. I personally prefer the clean look π
wow AJ! that was soo helpful.
Hi AJ,
Could you please help me understand how I now remove a button? In the previous versions, I had this:
What should I do now, in WP 3.9x?
Many thanks,
Bira
Hi Bira,
To be honest I haven’t looked into it. I don’t understand why you would possibly ever want to remove a button though…Have you looked into the Ultimate TinyMCE plugin? I’m pretty sure it lets you completely customize the tinyMCE in which case you can either use it or check out the code to see how they do it π
You can use array_diff() to remove buttons from a given row.
Hi AJ,
There are plenty of reasons you might want to remove a few buttons. As one, a client of ours believes that less is better and with the addition of TinyMCE 4.0, and all of the new features, there were a ton of buttons and menu items added to the TinyMCE editor.
We use multiple WYSIWYG editors in a CPT but don’t need any buttons other than bold, italic and the formatting buttons in that editor.
Thanks for this post by the way!
Hi AJ clarker,
How to add an multiple selectbox in editor.windowManager.open body, i just found a listbox type and cannot add a multiple selectbox in side this window.
Thanks
To be honest I haven’t had the need for it so I’m not sure if you can by default. You might have to create your own HTML for the pop-up window instead using the “URL” parameter. If you figure it out though, let me know! I don’t have a need for it yet, but maybe in the future!
It;s very very helpful. Thanks AJ.
Sweet– was using a lot of this functionality before. Learned the hard way. You have brushed some extra’s here.
Hey AJ,
Thanks for the great post. I was running in to an issue where I needed to add custom styles multiple times (once in my theme and once in a plugin). However, when I’d add two functions to the filter it would only add the styles from the last one added. I got around it by changing your code just a bit.
Became:
I know it is probably a one-off problem and there may be a more elegant way to handle it, but I thought I’d share what worked for me.
Thanks again!
I’m sure others will find it useful, thanks for sharing! Don’t forget though the WP coding standards (aka use curly brackets for if statements).
…and apparently I don’t know how to put code blocks into comments either. Happy Monday!
haha π For comments you can wrap it in the [ code] shortcode.
Hi,
I’ve seen that someone mentioned the font color palette. As anyone found the solution to have again the “more color” option that existed before? That was really handy…
Great post!
Kind regards
You could have a look at the code in this new plugin – TinyMCE Colorpicker – to see what the’ve done to bring it back.
Hey AJ thought you might be interested to see what I’ve done π We get a lot more power with the new TinyMCE so can do things like this:
https://wordpress.org/plugins/forget-about-shortcode-buttons/
Great looking plugin Ross! Thanks for sharing, I’ll be checking out your code π
Please do π I have some other cool ideas in the works leveraging this idea as well as some updates coming next week!
Has anyone found a way to change the label on the style_formats menu? (It’s “Formats” by default.)
I haven’t looked into it, but instead of renaming it, why not create your own dropdown?
Tnx fo the great article! I’m wondering if this could help me solve a LONG LONG LONG issue I had which is how to allow to use javascript (more specifically onclick events for Google Analytics) inside links. It seems that new TinyMCE invalidates all the solutions elsewhere available on the web…any idea?
Not so cool. It seems you cannot remove Visual and text quicktags without create a new tinyMCE object with wp_editor.
Thank you AJ!
This definitely saved me some time digging throught the TinyMCE API!
Hello AJ and all fellows over here, does anyone know how can I add link for label, here is a screenshot for the issue, hope it is clear enough.
Any help would be super duper appreciated π
i am having a error : can you suggest the possible problem i am having ??
ReferenceError: tinymce is not defined
i am using wp 3.9.1
Try de-activating your plugins 1 by 1, I think one of them might be adding some code that is conflicting with the tinymce. If you are using our Symple Shortcodes plugin, this could happen if you haven’t updated it.
thanks for this great tutorial, but in your wpex_mce_google_fonts_styles() function, how would I add multiple google font urls? Do I need to make a separate function for each?
I think you need to repeat the code for each link (not the function). Example:
Or at least that’s how I did it. And actually the way I did it was with an array, example:
Can you please let me know or some reference link of the different available “body -> type” parameter of ”
editor.windowManager.open” method.
Like i want to add html element, checkbox, text so on
Thanks
For futures, as it’s hard to find it even in TinyMCE manual…
http://www.tinymce.com/wiki.php/api4:namespace.tinymce.ui
thanks for the brilliant tutorial … exactly what I needed to get me start extending tinyMCE on wordpress … you have covered pretty much everything to get me started.
Hello AJ,
Thanks for this code snipet.
I want to add the custom dropdown with my own shortcode. I mean I can define my own shortcode in the .php or .js file and in the editor if I mouse over/click on the shortcode button then list appear and I will choose one shortcode.
List of shortcode like
[test1]Selected text[/text1]
[test2]Selected text[/text2]
[test3]Selected text[/text3]
Something like this.
I am trying to using your “Adding A Simple MCE Button” code snippet but its not allow me to select the shortcode.
Can you help me that how I can do this. Your help really help full for me.
Looking forward for your positive and quick reply.
Rajeev
Hi Rajeev, why don’t you take a look at my Symple Shortcodes plugin (it’s free) and see what I’ve done there.
AJ, I just installed your Symple Shortcodes plugin. Looks awesome and just what I’m looking for. Only problem is the little “S” icon is not showing up in the editor as shown in your YouTube tutorial. And I can’t figure out why. This is on WordPress 4.0.1.
Presumably is some setting I’ve missed somewhere. Any suggestions re: how to get the tools to show up would be much appreciated.
First make sure the version you are using is the latest one downloaded from here or the Github repositiory. Then clear any site/browser cache to make sure that’s not the issue. If you still don’t see it, de-activate 3rd party plugins incase one of them is breaking things.
Hello AJ,
Thanks for your prompt reply. But I have done with this by writing some jQuery rule and thanks for this lovely code snippet.
Rajeev
Great & useful, I’ve seen Symple Shortcodes, the problem is Pricing for example, I can’t submit the box because the popup window is hidden at the bottom. Even moving the box, not helpful. Scroll doesn’t exist. Am I the only one with this problem?
You might want to try re-downloading and installing it, because it should have a scrollbar on it.
AJ- I’m running into the same problem with the scrollbar not showing in the Pricing shortcode popup in Symple Shortcodes. I’m running the latest version of the plugin on a Mac…and don’t see the scrollbar on Safari, Chrome or FF.
I’m also trying to figure out how to get a scrollbar to show up for a separate shortcode popup that I’m building–it’s a rather large popup and won’t function without a scroll. Is there a parameter that needs to be set for TinyMCE?
I didn’t update the version number when I made the fix for the scrollbar, did you re-download and try updating and clearing the browser cache? I also use a Mac and it’s working fine. Have a look on Github what I did for the pricing module using autoscroll and giving it a fixed height and width.
Please resubmit your latest WordPress 3.9+ TinyMCE 4 Tweaks
Why? Everything still works the same.
Awesome! Just a note about a needed change that I ran into. When adding the JS file, for buttons for example, I had to use get_bloginfo( ‘stylesheet_directory’ ) instead of get_template_directory_uri() for the file path because I am using child themes (with genesis). Thought I’d mention it in case it is stumping someone else too!
Hi Karen,
Glad you found the post useful. Just a little heads up though, you really should use “get_stylesheet_directory_uri” instead of get_bloginfo( ‘stylesheet_directory’ ).
Ahh. Good point. I was moving too fast to think that through.
Hi AJ,
Thanks for this awesome TinyMCE lesson. But I want to add more things in pop-up window like my Own text, image, or anything.
How can I do this?
This works perfectly. Where could find a way to add an image uploader to the pop up ?
Thank you very much fir this article.
I have a question how can I display list of post categories on the Pop-Up Window?
Thanks
You’ll probably have to use wp_localize_script for this.
Awesome post ! Thanks AJ
Awesome stuff. Question: we have a user that cannot see the editor in on one of her computers using IE 10 i believe. Any idea what would cause this? We know its working for everyone else, just not here apparently.
Thanks
Have them clear their browser cache, 99% of the time this sort of thing is cache related (especially if it’s only happening for 1 user).
Hey AJ!
Just wanted to let you know that you just helped me develop my first private plugin for my sites. I had some issue getting it working at first, but it was just getting the call to the javascript file to the right location.
Regarding the location, I’d recommend anyone developing a basic plugin for their shortcodes to put the javacript with the plugin itself instead of in your theme area. That call is:
$plugin_array[‘my_mce_button’] = plugins_url( ‘/jsfilenamehere.js’, __FILE__ );
I’m glad to hear that Scott, awesome & thanks for the tip π
AJ, how would I go about changing “Sample Dropdown” to an icon? That’s about the only thing I’m having trouble understanding from the TinyMCE website.
It’s pretty simple, please read the section called “Add A Custom Icon To Your New MCE Button” in the above post π You can also have a look at my Symple Shortcodes plugin (also on github) and see how I did it there.
Hi Aj,
Good tutorial. This is very useful tutorial for WP developers. π
Hi there AJ,
Thank you for this wonderful post. It’s great to have such updated information that works!
I have everything working perfectly except for one thing.
I have added custom div tags and text through my popup using onsubmit function( e ).
This displays my block and text using custom css perfectly.
However after inserting, when I press return, I want it to start a new paragraph,
Instead it duplicates the previous div element and puts my next line of text in that.
Is there any way I can stop tinyMCE from replicating the previous block element?
Sorry Shane, I am not sure why that’s happening I would have to look at the whole code to see what you’ve done.
Hi Aj,
is it possible to add a image uploader in TinyMCE button? if possible then how?
I’m sure it’s possibly and not very hard, but I haven’t messed with that yet. Sorry.
Hi AJ! Can you help me?
In adminPanel i change styles. Styles is work good. But in blocks “span” not all style formats see.
For example color is red, but in span from Formats is black? Why? in WP 3.3 it works good. Now it’s broken?
See link with picture: pikucha.ru/idOBP
Thanks!
This is on purpose, you shouldn’t really add any styles to the drop-down list anyway, it’s much more user-friendly if they all look the same, just use names that describe what it is.
hi
how can i add a long list to listbox via array?
Use wp_localize_script that way you can access the array via the javascript and make use of it.
Hi there,
I added all your snippets to my custom theme’s function file, but nothing has changed at all when looking at the edit post page. Does the admin area have it’s own function file? Can’t get the new button to show up at all. Not worried about css/icons yet.
Assuming your WordPress installation is up to date and everything was copied correctly it should work. These are the exact snippets used to generate the screenshots and there hasn’t been any changes to the way WordPress works. Make sure you have opened your php tag in functions.php and also that you don’t have any errors (enable WP_Debug).
Hi,
according to this perfect manual I have made a plugin with my shortcodes. Ican’t reach the button in Black Studio Tiny Widget. By post or page visual editor I can see my registered button, but in widget (black studio tiny mce) it is not possible. Any idea what to add to see my button also in widget?
Many thanks!
I am not familiar with “Black Studio Tiny Widget”. If it’s a plugin, I suggest reaching out to the plugin developer to see if they can assist.
Typography play an important role in displaying your content in a better way. Adding more font sizes in WordPress is not a difficult job, all you need to install a plugin or insert some codes in CSS Editor.
Seriously awesome tutorial. Thanks for posting this AJ!
You’re awesome!!! Thank you for this.
The cool one! I want to customize my TinyMCE. Thanks!
Hi,
I want to get the value in the listbox but it always return text value (Yes/No instead of True/False). How can I do that? Thank you for awesome tutorial.
Great Tutorial!!!
Could the button were adding here be added also in the front end (let’s say when i’m using a rich text form field)?
Thanks.
Super super super helpful post-!! I do have one question – is it possible to make the “textbox” field in a dialog window into a WYSIWYG?
Thanks for this Superb Tutorial….
This is a great tutorial; The way you progressively made it more complex but with each phase producing its own end product was really well done. Thank you!
Certainly saved probably 8-10 hours of my research time π
Hey. Thanks for great article. Have just one question. Is it possible to change code so it wrap selected text in shortcode ? Thanks
How can I add date picker and color picker in popup?
I don’t know off the top of my head…but if you figure it out please share with others!
Great article and handy resource. Thanks!
AJ thank you so much for share this! You are my hero! π
Hi, thanks for that.
I’ve tried to add 13px font size copying the code you shared but it didn’t work.
There is this code and I’ve added 13px which didn’t work either. Then I deleted this code and only put yours but it doesn’t change anything.
Can you tell me what I’m doing wrong please?
Thanks
Did you make sure to add the “mce_buttons_2” filter first see under the section titled “Adding Font Size & Font Family Selects”. You need to make sure the font-selector exists first so that you can add the font sizes. I double checked and my code is correct. If still have issues make sure your WordPress installation is up to date and that you don’t have any plugins breaking things.
Thanks! It has quite some depth. My tinymce popup has improved. good insights.
Okay, I’m a year and a half late, but this is an outstanding post. Thank you!
Wow, this is an amazing and informative post! I want to add little information that should be known by every user who is using TinyMCE.
Itβs difficult to copy/paste the data to the editor from Word document, Excel, Powerpoint, or paint and you need a plugin to do this. I am using Pasteitcleaned and this plugin works fine with amazing functionality.
How can I create repeater field. Can you help me.?
Honestly – this is a pretty old guide and now days it would probably be better to create a Gutenberg block rather than a shortcode as explained in this post.