How to Create a WordPress Shortcode
WordPress shortcodes are used to output dynamic PHP based code anywhere on your site and are especially useful in areas such as post content, widgets, menus and Customizer settings. In this tutorial I’ll explain what shortcodes are, how to use them, how they are different from blocks, how to create your own shortcodes and provide you with an abstract class for registering multiple shortcodes.
If you are here just to learn how to create shortcodes you can skip to the code.
What are Shortcodes?
A shortcode is a WordPress function that is used to output complex and or dynamic code from your theme or a plugin via text added to your site.
According to WordPress.com they are “code that let’s you do nifty things with very little effort”. Which I guess is true, but not really a good definition. I like to think of shortcodes as aliases to PHP functions that can be used outside of PHP.
Shortcodes are written inside two square brackets:
[shortcode_name]
Shortcodes support optional attributes as such:
[shortcode_name attribute_1="value" attribute_2="value"]
Shortcodes may support inner content:
[shortcode_name]Some content[/shortcode_name]
Lastly, shortcodes can be nested inside each other:
[shortcode_group][shortcode_item][/shortcode_group]
Using Shortcodes in WordPress
Shortcodes can be added pretty much anywhere in WordPress including pages, posts, menu items, widgets and even custom code. Some areas don’t accept shortcodes by default but adding support is possible via hooks – let me know in the comments if you need help enabling support for shortcodes somewhere.
Adding Shortcodes in Gutenberg (Posts/Pages)
To add a shortcode inside a post you’ll use the appropriately named “Shortcode” block in Gutenberg. Simply insert the block then inside the field add your shortcode like such:
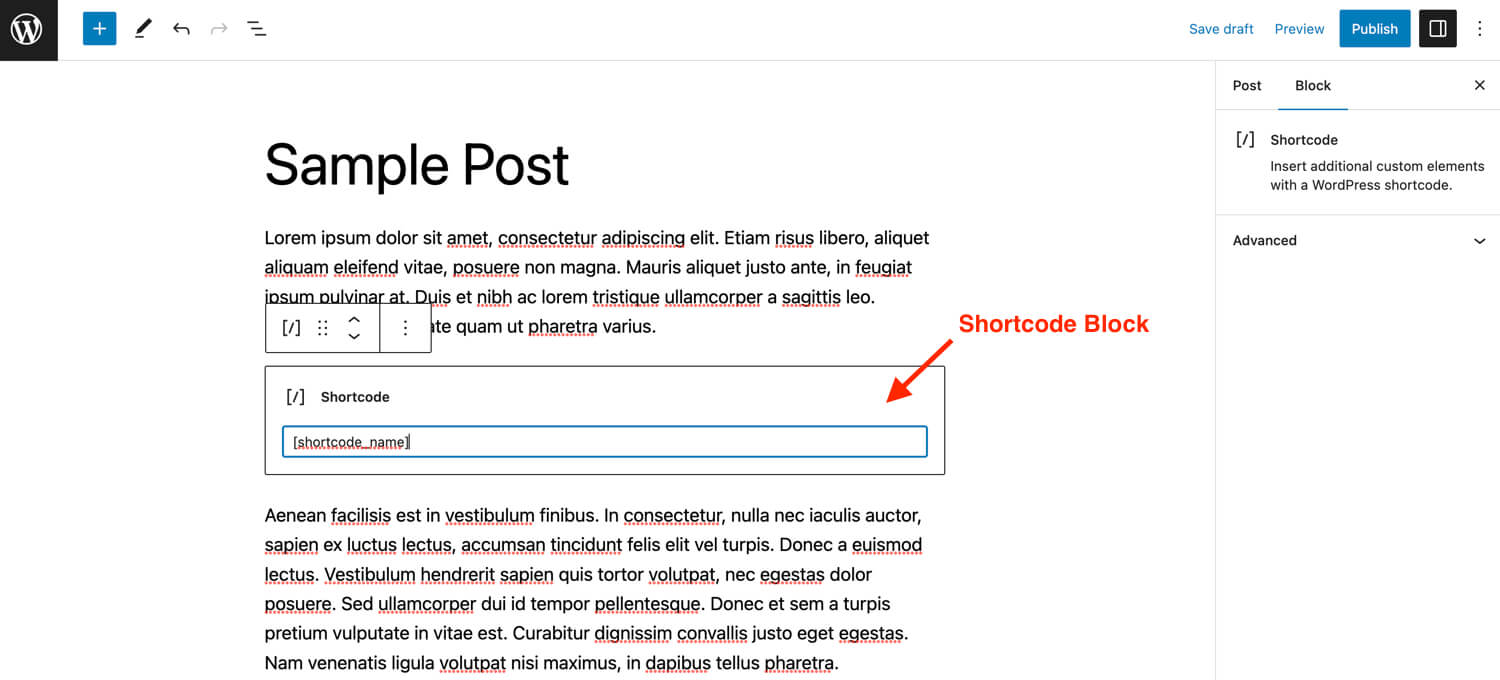
And of course if you are using the classic editor instead of Gutenberg you would just add it directly into the text editor.
Displaying Shortcodes inside your Theme or Plugin via Code
It’s also possible to use shortcodes inside your themes or plugins using PHP. Sometimes there may be a shortcode provided by a plugin that you want to use inside your theme.
You could search for a shortcode’s callback function and use it or you can simply wrap your shortcode inside the do_shortcode()
function like such:
<?php echo do_shortcode( '[shortcode_name]' ); ?>
I recommend using do_shortcode() instead of the callback function because the shortcode name should never change but developers often modify their code so if you use the callback function you may need to update your code in the future if anything changes.
Shortcodes vs Blocks
WordPress has switched over to a new “block” based system via Gutenberg so you may be wondering why would anyone even care about shortcodes. While blocks are similar to shortcodes in their purpose they are very different in the way they are created and used so shortcodes still have their place.
The main difference between blocks and shortcodes is that blocks may or may not be “dynamic” whereas shortcodes are always dynamic. This means that you can always control the output of a shortcode and the changes will be automatically reflected on the live site, this is not always the case with blocks.
Blocks
Blocks are registered via PHP and json and may require multiple files (index.js, index.asset.php, block.json, block.php) for their registration and usage. Blocks become available in the Gutenberg editor for easily adding them to pages and modifying their output. Blocks are also used when developing modern WordPress themes that make use of the “site editor”.
Creating custom blocks is much more complex and you’ll need to spend some time learning about it as well as understand json and modern javascript (jsx). Leave this to the professionals.
Shortcodes
Shortcodes are registered via PHP by a singular function making them a quick and simple solution when you need to display code from your theme or plugin on your site. Shortcodes are basically the original “blocks” as they allowed you to output advanced code in the classic editor. Some popular page builders such as the WPBakery Page Builder are actually shortcode based.
Even though WordPress has introduced blocks, shortcodes are still very useful due to their simplicity and are widely used by themes and plugins (plus a lot of people dislike Gutenberg…but let’s not get into that).
Creating shortcodes requires basic PHP knowledge and only a few lines of code. They are great for quickly inserting dynamic content anywhere on your site.
Creating Shortcodes
Now that you know what shortcodes are, how they are used and how they differ from blocks the next step is to go ahead and create your first shortcode. To create a shortcode you will use the core WordPress add_shortcode
function.
Basic “Hello” Shortcode
Here is a simple [say_hello]
shortcode that will simply output the word “Hello”:
/**
* Register the [say_hello] shortcode.
*/
function wpexplorer_hello_shortcode() {
return 'Hello';
}
add_shortcode( 'say_hello', 'wpexplorer_hello_shortcode' );
See how easy it is to create shortcodes! With just a few lines of code you’ve now registered your shortcode and can insert it anywhere on your site. Next I’ll share some more advanced examples.
Notice that we are returning a value in our shortcode. If you use “echo” in your shortcode it will not display where you’ve inserted it but “randomly” on the page. Your shortcodes should always return not echo content.
Shortcode Attributes
You can create shortcodes that accept attributes so they can accomplish different tasks. Below is a shortcode that accepts a single attribute and based on it’s value outputs different text:
/**
* Register the [say_something] shortcode.
*/
function wpexplorer_say_something_shortcode( $atts = [] ) {
$atts = shortcode_atts( [
'text' => '',
], $atts );
return $atts['text'] ?? '';
}
add_shortcode( 'say_something', 'wpexplorer_say_something_shortcode' );
In this example we’ve created a shortcode named [say_something]
which will display the value of the “text” attribute. Sample usage:
[say_something text="Hello"]
And of course this shortcode will then display the word “Hello”.
Shortcode with Content
Sometimes you need to display content inside a shortcode that can’t be passed via a parameter because they contain a lot of text and/or HTML. If this is the case, you’ll want to register a shortcode that accepts the $content
parameter.
Here is an example of a shortcode with content:
/**
* Register the [alert] shortcode.
*
* @link https://www.wpexplorer.com/how-to-create-a-wordpress-shortcode/
*/
function wpexplorer_alert_shortcode( $atts = [], $content = '' ) {
return '<div class="alert">' . wp_kses_post( $content ) . '</div>';
}
add_shortcode( 'alert', 'wpexplorer_alert_shortcode' );
The snippet above adds a new [alert]
shortcode which can be used on your site like such:
[alert]This is an alert[/alert]
By creating an alert shortcode you are able to insert alerts anywhere on your site and control the HTML output globally via your shortcode. Awesome!
Creating a Shortcode with Content & Attributes
Now that you know how to create basic shortcodes, let’s create a more advanced shortcode that has both content and attributes.
The following is an [alert]
shortcode that allows you to pass on a “type” attribute:
/**
* Register the [alert] shortcode.
*/
function wpexplorer_alert_shortcode( $atts = [], $content = '' ) {
$atts = shortcode_atts( [
'type' => 'warning',
], $atts );
$type = ! empty( $atts['type'] ) ? sanitize_text_field( $atts['type'] ) : 'warning';
return '<div class="alert alert--' . esc_attr( sanitize_html_class( $type ) ) . '">' . wp_kses_post( $content ) . '</div>';
}
add_shortcode( 'alert', 'wpexplorer_alert_shortcode' );
So now you can you can use the shortcode to display different alerts like such:
[alert type="info"]Here is some info.[/alert]
[alert type="warning"]Be careful, I am a warning.[/alert]
[alert type="error"]Something wen't wrong with this alert.[/alert]
Based on the alert “type” the alert will have a different classname that you can then target with CSS to create different styles for each.
Creating a Shortcode Abstract Class
When registering many shortcodes, creating an abstract class can prevent having to repeat code each time a new shortcode is registered. Abstract classes also allow you to control all of the related shortcodes from a single class.
Here is a an example of an abstract class you can use for creating shortcodes:
/**
* Abstract class for registering shortcodes.
*/
abstract class Shortcode_Abstract {
/**
* Returns shortcode attributes.
*/
abstract public static function get_attributes();
/**
* Renders the shortcode.
*/
abstract public static function render( $atts, $content );
/**
* Registers our shortcode.
*/
public static function register() {
\add_shortcode( static::TAG, [ static::class, 'callback' ] );
}
/**
* Shortcode callback function.
*/
public static function callback( $atts, $content = null, $shortcode_tag = '' ) {
$atts = shortcode_atts( static::get_attributes(), $atts );
return static::render( $atts, $content );
}
}
Abstract class usage:
/**
* Alert Shortcode.
*/
class Alert_Shortcode extends Shortcode_Abstract {
/**
* Defines the shortcode tag name.
*/
public const TAG = 'alert';
/**
* Returns the shortcode attributes.
*/
public static function get_attributes() {
return [
'type' => 'warning',
];
}
/**
* Renders the shortcode.
*/
public static function render( $atts, $content ) {
$type = ! empty( $atts['type'] ) ? sanitize_text_field( $atts['type'] ) : 'warning';
return '<div class="alert alert--' . esc_attr( sanitize_html_class( $type ) ) . '">' . wp_kses_post( $content ) . '</div>';
}
}
Alert_Shortcode::register();
Using an abstract class and child classes for registering our shortcodes means we can place each shortcode class in its own file and use autoloading to load the files. This will keep your code well organized when registering many shortcodes.
Plus, if WordPress ever changes how shortcodes are registered you will only need to update your abstract class and not every child class.
Creating Shortcodes in WordPress is Awesome!
I personally love WordPress shortcodes because they are so easy to create and use. Blocks are cool, but they require a lot more time and effort even to register. Even the most simple block will require multiple files and an advanced understanding on how they work.
Let me know in the comments how you are using shortcodes and if you prefer them over blocks. I’d love to hear your thoughts!