WordPress Tutorial: How To Create A WordPress Theme from HTML (Part 1)
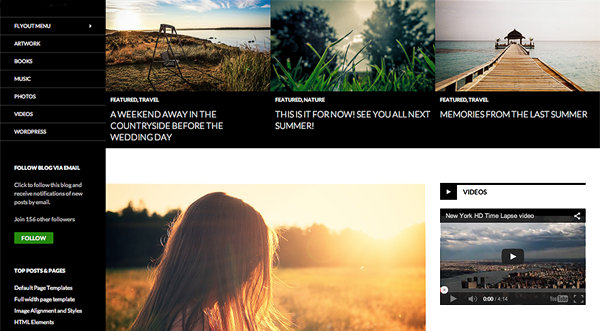
- 1. Currently Reading: WordPress Tutorial: How To Create A WordPress Theme from HTML (Part 1)
- 2. WordPress Tutorial: How To Create A WordPress Theme From HTML (Part 2)
- 3. An Intro to the Anatomy of a WordPress Theme
If you started with an HTML ( + CSS) website, you don’t have to throw it all away when moving to WordPress. You can convert your HTML into WordPress and run your (now more powerful) website on the dynamic WordPress platform.
Or maybe that’s not the case. Perhaps you are just wondering how to convert a client’s HTML design into a fully-fledged WordPress theme. Or maybe you would like to learn basic WordPress (+ PHP) programming from the more-familiar HTML side.
Whatever the reason you are here today, this WordPress tutorial will introduce you to the basics of creating a WordPress theme from HTML. You can follow this guide to create your theme from scratch or you can head over to Github and download the WPExplorer starter theme which provides an “empty canvas” to create your theme with all the necessary template files and code to get started. So if you are one of those people that rather learn by reading through code then download the starter theme, skip the guide and see how things work…Otherwise, get a code editor (I use and recommend Notepad++, or SublimeText) and a browser ready, then follow this simple guide to the end.
Naming Your WordPress Theme
First things first, we have to give your theme a unique name, which isn’t necessary if you’re building a theme for your website only. Regardless, we need to name your theme to make it easily identifiable upon installation.
General assumptions at this point:
- You have your index.html and CSS stylesheet ready.
- You have a working WordPress installation with at least one theme e.g. Twenty Fourteen
- You have already created a theme folder where you’ll be saving your new WordPress theme 🙂
Let’s get back to naming your WordPress theme. Open your code editor and copy-paste the contents of your stylesheet into a new file and save it as style.css in your theme folder. Add the following information at the very top of the newly-created style.css:
/*
Theme Name: Your theme's name
Theme URI: Your theme's URL
Description: A brief description of your theme
Version: 1.0 or any other version you want
Author: Your name or WordPress.org's username
Author URI: Your web address
Tags: Tags to locate your theme in the WordPress theme repository
*/
Do not leave out the (/*…*/) comment tags. Save the changes. This info tells WordPress the name of your theme, the author and complimentary stuff like that. The important part is the theme’s name, which allows you to choose and activate your theme via the WP dashboard.
Breaking Up Your HTML Template into PHP Files
This tutorial further assumes you have your HTML template arranged left to right: header, content, sidebar, footer. If you have a different design, you might need to play with the code a bit. It’s fun and super easy.
The next step involves creating four PHP files. Using your code editor, create index.php, header.php, sidebar.php and footer.php, and save them in your theme folder. All the files are empty at this point, so don’t expect them to do anything. For illustration purposes, I am using the following index.html and CSS stylesheet files:
INDEX.HTML
<!DOCTYPE html>
<head>
<meta charset="UTF-8">
<title>How To Convert HTML Template to WordPress Theme - WPExplorer</title>
<link rel="stylesheet" type="text/css" media="all" href="style.css"/>
</head>
<body>
<div id="wrap">
<header class="header">
<p>This is header section. Put your logo and other details here.</p>
</header><!-- .header -->
<div class="content">
<p>This is the main content area.</p>
</div><!-- .content -->
<div class="sidebar">
<p>This is the side bar</p>
</div><!-- .sidebar -->
<footer class="footer">
<p>And this is the footer.</p>
</footer><!-- .footer -->
</div><!-- #wrap -->
</body>
</html>
CSS STYLESHEET
#wrap{margin: 0 auto; width:95%; margin-top:-10px; height:100%;}
.header{width:99.8%; border:1px solid #999;height:135px;}
.content{width:70%; border:1px solid #999;margin-top:5px;}
.sidebar{float:right; margin-top:-54px;width:29%; border:1px solid #999;}
.footer{width:99.8%;border:1px solid #999;margin-top:10px;}
You can grab both codes if you have nothing to work with. Just copy-paste them into your code editor, save them, create the four PHP files we just mentioned and get ready for the next part. Open your newly-created (and empty) header.php. Login into your existing WordPress installation, navigate to Appearance –>> Editor and open header.php. Copy all the code between the <head> tags and paste it into your header.php file. The following is the code I got from the header.php file in Twenty Fourteen theme:
<head>
<meta charset="<?php bloginfo( 'charset' ); ?>">
<meta name="viewport" content="width=device-width">
<title><?php wp_title( '|', true, 'right' ); ?></title>
<link rel="profile" href="http://gmpg.org/xfn/11">
<link rel="pingback" href="<?php bloginfo( 'pingback_url' ); ?>">
<!--[if lt IE 9]>
<script src="<?php echo get_template_directory_uri(); ?>/js/html5.js"></script>
<![endif]-->
<?php wp_head(); ?>
</head>
Then open your index.html file and copy the header code (i.e. the code in the <div class= “header”> section) to your header.php just below the <head> tags as shown below:
<html>
<head>
<meta charset="<?php bloginfo( 'charset' ); ?>">
<meta name="viewport" content="width=device-width">
<title><?php wp_title( '|', true, 'right' ); ?></title>
<link rel="profile" href="http://gmpg.org/xfn/11">
<link rel="pingback" href="<?php bloginfo( 'pingback_url' ); ?>">
<link rel="stylesheet" href="<?php bloginfo('stylesheet_url'); ?>" type="text/css" />
<!--[if lt IE 9]>
<script src="<?php echo get_template_directory_uri(); ?>/js/html5.js"></script>
<![endif]-->
<?php wp_head(); ?>
</head>
<body>
<header class="header">
<p>This is header section. Put your logo and other details here.</p>
</header>
Then add…
<link rel="stylesheet" href="<?php bloginfo('stylesheet_url'); ?>" type="text/css" />
…anywhere between the <head> tags in the header.php file to link your stylesheet. Remember also to put the <html> and <body> opening tags in the header.php as shown above. Save all changes.
Copy the second section (i.e. <div class=”content”>…</div> from index.html to the newly-created index.php , and add…
<?php get_header(); ?>
…at the very top and …
<?php get_sidebar(); ?>
<?php get_footer(); ?>
…to the absolute bottom. These three lines fetch the header.php, sidebar.php and footer.php (in that order) and display them in the index.php, which puts your code back together. Save all changes. At this point, your index.php file should look like:
<?php get_header(); ?>
<?php get_sidebar(); ?>
<?php get_footer(); ?>
Then copy the content from the sidebar and footer sections in your index.html to sidebar.php and footer.php respectively.
Adding Posts
Your HTML template is about to morph into a WordPress theme. We just need to add your posts. If you have posts on your blog, how would you display them in your custom-made “HTML-to-WordPress” theme? You use a special type of PHP function known as the Loop. The Loop is just a specialized piece of code that displays your posts and comments wherever you place it.
Now, to display your posts in the content section of the index.php template, copy and paste the following code between the <div class=”content”> and </div> tags as shown below:
<div class="content">
<?php if ( have_posts() ) : ?>
<?php while ( have_posts() ) : the_post(); ?>
<div id="post-<?php the_ID(); ?>" <?php post_class(); ?>>
<div class="post-header">
<div class="date"><?php the_time( 'M j y' ); ?></div>
<h2><a href="<?php the_permalink(); ?>" rel="bookmark" title="Permanent Link to <?php the_title_attribute(); ?>"><?php the_title(); ?></a></h2>
<div class="author"><?php the_author(); ?></div>
</div><!--.post-header-->
<div class="entry clear">
<?php if ( function_exists( 'add_theme_support' ) ) the_post_thumbnail(); ?>
<?php the_content(); ?>
<?php edit_post_link(); ?>
<?php wp_link_pages(); ?>
</div><!--. entry-->
<footer class="post-footer">
<div class="comments"><?php comments_popup_link( 'Leave a Comment', '1 Comment', '% Comments' ); ?></div>
</footer><!--.post-footer-->
</div><!-- .post-->
<?php endwhile; /* rewind or continue if all posts have been fetched */ ?>
<nav class="navigation index">
<div class="alignleft"><?php next_posts_link( 'Older Entries' ); ?></div>
<div class="alignright"><?php previous_posts_link( 'Newer Entries' ); ?></div>
</nav><!--.navigation-->
<?php else : ?>
<?php endif; ?>
</div><!--.content-->
That will take care of your posts. Easy as ABC. At this juncture (and using the sample files provided in this tutorial), your header.php should look like this:
<head>
<meta charset="<?php bloginfo( 'charset' ); ?>">
<meta name="viewport" content="width=device-width">
<title><?php wp_title( '|', true, 'right' ); ?></title>
<link rel="profile" href="http://gmpg.org/xfn/11">
<link rel="pingback" href="<?php bloginfo( 'pingback_url' ); ?>">
<!--[if lt IE 9]>
<script src="<?php echo get_template_directory_uri(); ?>/js/html5.js"></script>
<![endif]-->
<?php wp_head(); ?>
</head>
Your sidebar.php should look like this:
<?php dynamic_sidebar( 'sidebar' ); ?>
Your footer.php should look like this:
<footer class="footer">
<p>And this is the footer</p>
</footer>
<?php wp_footer(); // Crucial core hook! ?>
</body>
</html>
Did you notice the closing </body> and </html> tags in the footer? Don’t forget to include those too.
Your style.css should look something like this:
/*
Theme Name: Creating WordPress Theme from HTML
Theme URI: http://wpexplorer.com
Description: This theme shows you how to create WordPress themes from HTML
Version: 1.0
Author: Freddy for @WPExplorer
Author URI: http://WPExplorer.com/create-wordpress-theme-html-1/
Tags: wpexplorer, custom theme, HTML to WordPress, create WordPress theme
*/
body {
font-family: arial, helvetica, verdana;
font-size: 0.8em;
width: 100%;
height: 100%;
}
.header {
background-color: #1be;
margin-left: 14%;
top: 0;
border-width: 0.1em;
border-color: #999;
border-style: solid;
width: 72%;
height: 250px;
}
.content {
background-color: #999;
margin-left: 14%;
margin-top: 5px;
float: left;
width: 46%;
border-width: 0.1em;
border-color: #999;
border-style: solid;
}
.sidebar {
background-color: #1d5;
margin-right: 14%;
margin-top: 5px;
float: right;
border-width: 0.1em;
border-color: #999;
border-style: solid;
top: 180px;
width: 23%;
}
.footer {
background-color: red;
margin-left: 14%;
float: left;
margin-top: 5px;
width: 72%;
height: 50px;
border-width: 0.1em;
border-color: #999;
border-style: solid;
}
And your index.php should look similar to:
<?php get_header(); ?>
<div class="content">
<?php if ( have_posts() ) : ?>
<?php while ( have_posts() ) : the_post(); ?>
<div id="post-<?php the_ID(); ?>" <?php post_class(); ?>>
<div class="post-header">
<div class="date"><?php the_time( 'M j y' ); ?></div>
<h2><a href="<?php the_permalink(); ?>" rel="bookmark" title="Permanent Link to <?php the_title_attribute(); ?>"><?php the_title(); ?></a></h2>
<div class="author"><?php the_author(); ?></div>
</div><!--.post-header-->
<div class="entry clear">
<?php if ( function_exists( 'add_theme_support' ) ) the_post_thumbnail(); ?>
<?php the_content(); ?>
<?php edit_post_link(); ?>
<?php wp_link_pages(); ?>
</div><!--. entry-->
<footer class="post-footer">
<div class="comments"><?php comments_popup_link( 'Leave a Comment', '1 Comment', '% Comments' ); ?></div>
</footer><!--.post-footer-->
</div><!-- .post-->
<?php endwhile; /* rewind or continue if all posts have been fetched */ ?>
<nav class="navigation index">
<div class="alignleft"><?php next_posts_link( 'Older Entries' ); ?></div>
<div class="alignright"><?php previous_posts_link( 'Newer Entries' ); ?></div>
</nav><!--.navigation-->
<?php else : ?>
<?php endif; ?>
</div><!--.content-->
<?php get_sidebar(); ?>
<?php wp_footer(); // Crucial footer hook! ?>
<?php get_footer(); ?>
Again – this is based on a left to right website with a header, content, sidebar, footer layout. Are you following? All the five files should be saved in your theme folder. Name the folder whatever you want and compress it into a ZIP archive using WinRar or an equivalent program. Upload your new theme to your WordPress installation, activate it and see your converted theme in action!
That was easy, right? You can style your theme however you want but most of the features we love in WordPress are still inactive since…this tutorial covers the basics of converting HTML templates into WordPress and should be of great value to you as a beginner. In the next tutorial, we will take things a notch higher and play around with other aspects of WordPress programming (such as Template Files and Template Tags) that will let you turn your HTML templates whichever way you like. Stay tuned!
Also don’t forget to add wp_footer() immediately before the tag in your footer.php.
Yep, very important will update the post now!
Great Tutorial, I still have to try it though but great work guys.
Thank you for your comment Hann. Please do try and let us know 🙂
Hi this is a nice tutorial regarding wordpress theme development
Glad you liked it Manoj!
This is a great tutorial..i am new to wordpress and i am sure with this site i will become an expert. Thank you very much.
Placidius
I have really got something here. This is nice Freddy. But, will my theme that has the php code for “POSTS” embedded in the html template work when converted into a wordpress theme?
Thank you for your comment Premo! If you can, import your posts after converting the template into a theme. WordPress supports different formats when importing, so you ought to be covered. Hope that helps 🙂
Great post, but please use some white space next time. That code can blind you trying to read it.
Ha you are correct, these snippets need to be properly formatted, thanks for the heads up! I will keep a closer eye on that.
great post about wordPress. I would like to thank to the author for such a nice post
You’re far too kind Vidhya! Thanks for passing by and sharing 🙂
I so I am very new to wordpress and am finding i prefer html, but have been asked to put my site on wordpress, if i have posts in my side bar do i have to put a loop there too. I am quite confused. Please help
Yes, if you want posts on your sidebar the best thing is to create a custom widget and use WP_Query to loop through the posts. But there are already a ton of great recent posts widget plugins out there you could probably use instead of developing your own from scratch.
great tutorial for beginners…
Great tutorial, recently I have found another tutorial series in which the author teaches on how to convert any HTML business theme to WordPress. The techniques in this tutorial are applicable on any HTML busienss theme we want to work with. Here is the source: http://www.cloudways.com/blog/convert-html-to-wordpress-business-theme/
Thanks for sharing! That’s a great article as well – and it’s by one of our other awesome authors, Ahsan 🙂
From last few days, I am trying to convert my website from HTML to WordPress CMS…But unfortunately, it doesn’t happen yet…After reading your blog, I get to learn more about the conversion and found that its the simplest method mentioned in a easiest way…Thanks for sharing the useful post with us…
Thank you Mike for the input. It’s much appreciated. Good luck with the conversion, and if you ever get stuck, I can always help with a few tips 🙂
Nice Work.. (y) but please display code in more readable form (by formatting it).
Good idea, I will clean up the code right now!
Great tutorial
Thanks Abdul!
Awesome tutorial for beginners in WP like me. Thanks. 🙂
Thank you Sanya for passing by and the kind words. You’re going to have an awesome time with WordPress 🙂
Just what I was looking for.. Thank you Freddy 🙂
You are welcome Raj. Thank you for contributing…
I’m having very simple VCard HTML template and almost zero knowledge about coding.Did it as per tutorial but when i upload to WP,theme is recognized but blank.No content at all.
Only thing i didn’t add to those files is part about blog posts,as it ain’t blog.
Any idea what might be the problem?
Thanx!
Try enabling WP_Debug on your server to see if you have any sort of PHP error.
Thanks for the nice tutorial. I am new to wordpress.This helped me a lot.
Hey Aliya 🙂 You’re welcome. You will have a great time with WordPress! See you around.
Great Tutorial
Glad you loved it Oscar. Thank you for passing by 🙂
thanks man it has helped me
Jambo Mwendwa!
Thank you for passing by and commenting 🙂 Your input is much appreciated!
Great Tutorial
Thanks for the nice tutorial. I am new to wordpress.This helped me a newcreate a theme
That’s the spirit 🙂 Thank you for reading and sharing with us 🙂
hello, thank yo for great tutorial. How to integrate javascript slider on the Homepage ? Like parallax feel to it?
I would recommend using a plugin such as Revolution Slider.
I have a doubt! Why the css I put for my theme is not applied in internet explorer! It worked in mozilla and chrome. For example the border-radius css not applying in internet explorer. If IE is not compatible for that is there is a way around for it!
CSS3 only works on the latest version of Internet Explorer. There isn’t a way around that (not any good way). People need to update their browsers 😉
Nice tutorial this will really help for theme integration
Awesome 🙂
really great tutorial it help me so much how to make wp-theme
Thanks Mubashar 🙂
Awesome. I am going to try it out.
THANKS FOR TUTORIAL
Great Tutorial.Thanks
Its a great tutorial! Helped me a lot!
Thanx man!
You’re far too kind. Thanks for passing by!
This is really an informative & useful post. But,I also heard about a software TemplateToaster which is easy to use and compatible with almost all CMSs like wordpress, joomla,drupal etc.I have been used this tool for creating wordpress theme and I am happy to use this tool as it diminished the coding process.
this is awesome tutorial.thanks alot
I was searching for WordPress theme development and got your article there in Google.
I am a newbie in this development field and will try your method for sure.
Can i ask my queries direct here in future?
You sure can 🙂 Thanks for contributing!
Thanks for sharing information about creation Of WordPress theme.
It is very helpful post .
plz tell me my theme does not have widget . and want to ask what is the use of function.php
Functions.php is the main file used to add all the main theme functions or to include all the necessary function files for the theme. To add a widget area you will have to use the register_sidebar function.
such a nice tutorial freddy
Thanks for the kind words Hassan 🙂
THank you soooo much freddy. It was so much usefull.
You’re welcome Piyush!
That’s exactly what I was looking for! Really really helpful! Thank you for writing and sharing! ;D
Awesome! I’m so glad the post was helpful 🙂 Thank you for passing by!
Thanks for such easy steps how to make wordpress theme
You’re welcome Vijay…thanks for sharing your thoughts 🙂
This is a very nice post. You r a good tutor . please brief discribe it on next post
You’re far too kind Arun. Thanks for passing by 🙂 Happy Holidays!
Great tutorial but the sidebar.php , i think it’s wrong .
Hey Freddy,
I have been using WordPress for many years but never tried to make even a basic WP theme. I’ll try this tutorial this weekend. I’d appreciate it if you guide me further on putting Two sidebars on WordPress theme. One on the left and One on the right.
Thanks!
Awesome Plaintips…go ahead and give it a shot. I’m sure you’ll love it 🙂 Thank you for passing by!
Good TUtorial and well starter to the begineers sure i will follow those steps
Awesome!
Just what i was looking for! Thank u Freddy!
Thank you Nikhil for contributing!
NIce job on this tutorial. I will start converting my html version tot WP now. Thnx!
Thank you for the compliment…have fun and don’t forget to share what you learn 🙂
Thnx Bro it reall helps me..
Get work Freddy!
Thank you for passing by Freddy 🙂
Great Post! Thanks for sharing great knowledge… Appreciate
My pleasure Faisal. Thank you for the kind words and your much appreciated input!
very simple and great post for the beginners
I am new in wordpress. Nice tutorial. Thank you for sharing nice post. after submitting the registration form i want to store the data to database in wordpress. without plugin how to do it?
Hey Ananth, you just need WordPress and a membership plugin. The rest is automatic 🙂 Hope that helps!
Thanks for Your tutorial……… please continue it………… 🙂
Thank you passing by Ahmed. You can read part 2 here: WordPress Tutorial: How To Create A WordPress Theme From HTML (Part 2) Enjoy!
Very helpful tutorial. Thanks for sharing this important information.
this is very helpful article!! lerned a lot from it!!Thanks
Thanks to Share useful article i am new too wordpress . I find this post very useful for make my theme .
I meet the error WordPress: Call to undefined function get_header(),get_header() run in index.php but this not run in amthuc.php
could you help me?
If you get the error undefined function get_header() this means your WordPress installation isn’t installed, or not installed correctly, or missing core files. This function is a core function so it should definately be defined.
If you get the error undefined function get_header() this means your WordPress installation isn’t installed, or not installed correctly, or missing core files. This function is a core function so it should definitely be defined.
Hi there,
Great tutorial. It helped me a lot in learning the basic website building and designing tips.
Oh yeah! I like that you learned something Daniel. Keep it rocking, and thank you for the compliment 🙂
Amazing steps shared.I didnt know that wordpress theme can be created in such easy steps and following this.
That’s the beauty of WordPress Spencer…it’s easy, easy ….eaaasy Thank you for passing by 🙂
THANK YOU FOR yOUR TUTORIAL.
This is a great tutorial.
If there are any more tutorials by you on wordpress please inform me ——– Freddy.
Freddy Thank you for your great support.
You’re welcome Pavan! Keep it WPExplorer for more WordPress tutorials, tips and much much more.
What about the navigation?
WordPress navigation/menus are created using by first registering your menu with register_nav_menus then displaying them using wp_nav_menu.
hey thank you for sharing this post. Nice one.
Thank you for your comment Amit. Much appreciated 🙂
You are a HERO Freddy, THANK YOU SO MUCH, over simplify is an art that most developers lack when it comes to teach absolute beginners what they already know, and you mastered that skill.
This is a great comment Ulises Calvo! Means a lot to yours truly; the compliment is much appreciated. Thank you for taking the time.
good collections
Awesome 🙂 Thanks for the comment!
great post till now i avoiding to make wordpress theme, right now i m making it great!!
Isn’t that just awesome Salmankhan? I’m glad you’re having fun making WordPress themes. I wish you all the best in your future endeavors! Thank you for contributing 🙂
Thanks for the step-by-step tutorial. even though it seems really clear, im having problems with uploading my newly created theme as zip file.
I get the following warning when adding my new theme:
Warning: Invalid argument supplied for foreach() in /Applications/XAMPP/xamppfiles/htdocs/wordpress_sterre_carron/wp-includes/update.php on line 311
Warning: Invalid argument supplied for foreach() in /Applications/XAMPP/xamppfiles/htdocs/wordpress_sterre_carron/wp-includes/update.php on line 315
Unable to create directory wp-content/uploads/2015/11. Is its parent directory writable by the server?
I’ve already put an extra line to debug since im using localhost but i don’t see what im doing wrong in here.. Maybe someone can help me?
thanks in advance!
Ok i already fixed it by placing the style.css directly in my new theme folder.
But now there’s a likely problem; when i want to install a new plugin i get the error unable to create directory..
Maybe someone can help me to fix this? Ive already searched through different fora but there wasn’t a right solution
This error is actually server related. You have to fix server permissions for this, I would suggest contacting your webhost for assistance, in most cases they will help you out. We use WPEngine which doesn’t have these problems by default, but some shared hosts will require you to chown down or chmod permissions on your server after installing WordPress.
Great Tutorial Buddy… It helps me a lot.. But something I want to know that.. As we create header.php, index.php, sidebar.php, footer.php there are lots of other pages also in those three pre themes. I want to know about all pages which are in twenty-twelve theme, like function.php, search.php and all other…. please help me out.. thanks
Hi Freddy !! Great Tutorial for WordPress Beginners.. Please keep it up for advanced !!
it’s really good thing. i like it.
You have provided quite an informative tutorial on wordpress theme on html.I was having some doubts regarding this.Now I think your post will help me to solve my issues.
Thanks
You said in the beginning, you have to make a folder, but where has the folder to be?? Inside the wordpress zip or something? Or do you have to but it in filezilla (program I use)???
This depends where your WordPress installation is located or if you want to create your theme then upload it. You could add it to your WordPress site at root/wp-content/themes/ and then add your files as you create them for the theme. Or you can simply create the folder on your computer and add all your template files without testing and once finished upload it to WordPress either via FTP or zip it up and install it in WordPress at Appearance > Themes > Add New.
The best tutorial so far I have seen ….
thanks to tutorials like yours I was able to make the first version for WordPress of my theme generator available on template-creator.com
So thank you!
Wow, that’s what I was exploring for, what a information! existing here at this website, thanks admin of this site.
nice one, i will try it.
Thanks , nice Article , really helpful .
Nice Informative Article.
You did a great job here. I like the way you represented it.
Thanks for sharing.
Hi Freddy,
Thanks so much for this tut. I was just trying to figure out how to phrase my question correctly to get the right information when I found it this morning. Have you considered making this a video & putting it up on youtube? A bit of background – I started using WordPress 2 yrs ago, trying to help out a friend build a blog. Fast forward 12 months, after intro to Dreamweaver & loving it, looked back at the blog after steep learning curve on WP and realised it’s a bit of a mess. I want to create my own themes, & while no issue with a ‘normal’ website, wasn’t sure how to tackle the posts side of things – getting them rolling so latest is top etc.
I’m still plugging away, learning php, etc., but want to get my friends blog happening asap. This is exactly what I needed.
Thanks again.
is the class like “content” or “post-header” come from wordpress or we can make that as we wish to make ?
It can be anything you want. When creating a WordPress theme you can use any classnames you want in your code, however, even though we didn’t do it in this Tutorial it is recommended that you prefix all your classnames. So for example instead of using id=”header” you would use id=”prefix-header” where “prefix” is any prefix you decide, I personally use “wpex” as the prefix for most my themes (short for WPExplorer). By using prefixes you avoid any potential conflicts with 3rd party plugins.
Pls, i’m working on a WordPress theme, But i dont know how to link single.php from index.php..
i need help on that… thanks in advance
You don’t link to single.php. The single.php file is the file that gets automatically loaded by WordPress whenever someone visits a single post.
i am planning to conver my HTMl design for one website to convert it to WordPress using this method can i conver this all and what about images and all you havnt mention here about it.
please guide I am not expert programmer but it will help me
Images used in your design would still be placed in the same location. In the CSS file you would still use relative locations so there is nothing different there. Images that may be used in the design itself (maybe like a banner or logo) you should already have these in some sort of folder in your theme and instead of linking to them manually ore relatively you should be using the get_template_directory_uri to link to your theme folder on the server.
Hello,
Thanks for sharing such informative and helpful blog post to create my first theme. You are doing a good job so keep posting such amazing articles for learning WordPress.
Thank you, very helpful, from the few articles I’ve read, this is the most easily understood.