My 25 Best WooCommerce Snippets For WordPress
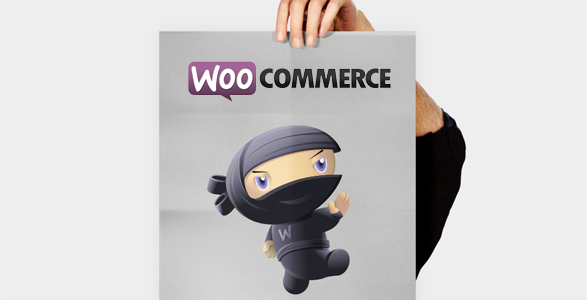
- 1. Currently Reading: My 25 Best WooCommerce Snippets For WordPress
- 2. My 25 Best WooCommerce Snippets For WordPress Part 2
Times flies… It’s been 6 months since I started to work for WooThemes as a WooCommerce full time support technician. During these 6 months I learnt a lot on WooCommerce and I coded a lot (read a lot!) of WooCommerce related snippets. Actually, WooCommerce is really powerful tool and easily expandable. It has many hooks that can be used for nearly everything, and that’s what make WooCommerce so good. Here is a list of snippets I wrote or adapted during the past months; all these snippets must be pasted in the functions.php file within your theme folder:
1 – Add Payment Type to WooCommerce Admin Email
add_action( 'woocommerce_email_after_order_table', 'add_payment_method_to_admin_new_order', 15, 2 );
function add_payment_method_to_admin_new_order( $order, $is_admin_email ) {
if ( $is_admin_email ) {
echo '<p><strong>Payment Method:</strong> ' . $order->payment_method_title . '</p>';
}
}
2 – Up-sells products per page / per line
remove_action( 'woocommerce_after_single_product_summary', 'woocommerce_upsell_display', 15 );
add_action( 'woocommerce_after_single_product_summary', 'woocommerce_output_upsells', 15 );
if ( ! function_exists( 'woocommerce_output_upsells' ) ) {
function woocommerce_output_upsells() {
woocommerce_upsell_display( 3,3 ); // Display 3 products in rows of 3
}
}
3 – Remove product categories from shop page
add_action( 'pre_get_posts', 'custom_pre_get_posts_query' );
function custom_pre_get_posts_query( $q ) {
if ( ! $q->is_main_query() ) return;
if ( ! $q->is_post_type_archive() ) return;
if ( ! is_admin() && is_shop() && ! is_user_logged_in() ) {
$q->set( 'tax_query', array(array(
'taxonomy' => 'product_cat',
'field' => 'slug',
'terms' => array( 'color', 'flavor', 'spices', 'vanilla' ), // Don't display products in these categories on the shop page
'operator' => 'NOT IN'
)));
}
remove_action( 'pre_get_posts', 'custom_pre_get_posts_query' );
}
4 – Quickly translate any string
add_filter('gettext', 'translate_text');
add_filter('ngettext', 'translate_text');
function translate_text($translated) {
$translated = str_ireplace('Choose and option', 'Select', $translated);
return $translated;
}
5 – Exclude a category from the WooCommerce category widget
add_filter( 'woocommerce_product_categories_widget_args', 'woo_product_cat_widget_args' );
function woo_product_cat_widget_args( $cat_args ) {
$cat_args['exclude'] = array('16');
return $cat_args;
}
6 – Add a custom field to a product variation
//Display Fields
add_action( 'woocommerce_product_after_variable_attributes', 'variable_fields', 10, 2 );
//JS to add fields for new variations
add_action( 'woocommerce_product_after_variable_attributes_js', 'variable_fields_js' );
//Save variation fields
add_action( 'woocommerce_process_product_meta_variable', 'variable_fields_process', 10, 1 );
function variable_fields( $loop, $variation_data ) { ?>
<tr>
<td>
<div>
<label></label>
<input type="text" size="5" name="my_custom_field[]" value=""/>
</div>
</td>
</tr>
<tr>
<td>
<div>
<label></label>
</div>
</td>
</tr>
<?php }
function variable_fields_process( $post_id ) {
if (isset( $_POST['variable_sku'] ) ) :
$variable_sku = $_POST['variable_sku'];
$variable_post_id = $_POST['variable_post_id'];
$variable_custom_field = $_POST['my_custom_field'];
for ( $i = 0; $i < sizeof( $variable_sku ); $i++ ) :
$variation_id = (int) $variable_post_id[$i];
if ( isset( $variable_custom_field[$i] ) ) {
update_post_meta( $variation_id, '_my_custom_field', stripslashes( $variable_custom_field[$i] ) );
}
endfor;
endif;
}
7 – Replace “Out of stock” by “sold”
add_filter('woocommerce_get_availability', 'availability_filter_func');
function availability_filter_func($availability)
{
$availability['availability'] = str_ireplace('Out of stock', 'Sold', $availability['availability']);
return $availability;
}
8 – Display “product already in cart” instead of “add to cart” button
/**
* Change the add to cart text on single product pages
*/
add_filter( 'woocommerce_product_single_add_to_cart_text', 'woo_custom_cart_button_text' );
function woo_custom_cart_button_text() {
global $woocommerce;
foreach($woocommerce->cart->get_cart() as $cart_item_key => $values ) {
$_product = $values['data'];
if( get_the_ID() == $_product->id ) {
return __('Already in cart - Add Again?', 'woocommerce');
}
}
return __('Add to cart', 'woocommerce');
}
/**
* Change the add to cart text on product archives
*/
add_filter( 'add_to_cart_text', 'woo_archive_custom_cart_button_text' );
function woo_archive_custom_cart_button_text() {
global $woocommerce;
foreach($woocommerce->cart->get_cart() as $cart_item_key => $values ) {
$_product = $values['data'];
if( get_the_ID() == $_product->id ) {
return __('Already in cart', 'woocommerce');
}
}
return __('Add to cart', 'woocommerce');
}
9 – Hide products count in category view
add_filter( 'woocommerce_subcategory_count_html', 'woo_remove_category_products_count' );
function woo_remove_category_products_count() {
return;
}
10 – Make account checkout fields required
add_filter( 'woocommerce_checkout_fields', 'woo_filter_account_checkout_fields' );
function woo_filter_account_checkout_fields( $fields ) {
$fields['account']['account_username']['required'] = true;
$fields['account']['account_password']['required'] = true;
$fields['account']['account_password-2']['required'] = true;
return $fields;
}
11 – Rename a product tab
add_filter( 'woocommerce_product_tabs', 'woo_rename_tab', 98);
function woo_rename_tab($tabs) {
$tabs['description']['title'] = 'More info';
return $tabs;
}
12 – List WooCommerce product Categories
$args = array(
'number' => $number,
'orderby' => $orderby,
'order' => $order,
'hide_empty' => $hide_empty,
'include' => $ids
);
$product_categories = get_terms( 'product_cat', $args );
$count = count($product_categories);
if ( $count > 0 ){
echo "<ul>";
foreach ( $product_categories as $product_category ) {
echo '<li><a href="' . get_term_link( $product_category ) . '">' . $product_category->name . '</li>';
}
echo "</ul>";
}
13 – Replace shop page title
add_filter( 'woocommerce_page_title', 'woo_shop_page_title');
function woo_shop_page_title( $page_title ) {
if( 'Shop' == $page_title) {
return "My new title";
}
}
14 – Change a widget title
/*
* Change widget title
*/
add_filter( 'widget_title', 'woo_widget_title', 10, 3);
function woo_widget_title( $title, $instance, $id_base ) {
if( 'onsale' == $id_base) {
return "My new title";
}
}
15 – Remove WooCommerce default settings
add_filter( 'woocommerce_catalog_settings', 'woo_remove_catalog_options' );
function woo_remove_catalog_options( $catalog ) {
unset($catalog[23]); //Trim zeros (no)
unset($catalog[22]); //2 decimals
unset($catalog[21]); //decimal sep (.)
unset($catalog[20]); //thousand sep (,)
unset($catalog[19]); //currency position (left)
unset($catalog[18]); //currency position (left)
unset($catalog[5]); // ajax add to cart (no)
return $catalog;
}
16 – Change “from” email address
function woo_custom_wp_mail_from() {
global $woocommerce;
return html_entity_decode( 'your@email.com' );
}
add_filter( 'wp_mail_from', 'woo_custom_wp_mail_from', 99 );
17 – Decode from name in WooCommerce email
function woo_custom_wp_mail_from_name() {
global $woocommerce;
return html_entity_decode( get_option( 'woocommerce_email_from_name' ) );
}
add_filter( 'wp_mail_from_name', 'woo_custom_wp_mail_from_name', 99 );
function woo_custom_wp_mail_from() {
global $woocommerce;
return html_entity_decode( get_option( 'woocommerce_email_from' ) );
}
add_filter( 'wp_mail_from_name', 'woo_custom_wp_mail_from_name', 99 );
18 – Return featured products ids
function woo_get_featured_product_ids() {
// Load from cache
$featured_product_ids = get_transient( 'wc_featured_products' );
// Valid cache found
if ( false !== $featured_product_ids )
return $featured_product_ids;
$featured = get_posts( array(
'post_type' => array( 'product', 'product_variation' ),
'posts_per_page' => -1,
'post_status' => 'publish',
'meta_query' => array(
array(
'key' => '_visibility',
'value' => array('catalog', 'visible'),
'compare' => 'IN'
),
array(
'key' => '_featured',
'value' => 'yes'
)
),
'fields' => 'id=>parent'
) );
$product_ids = array_keys( $featured );
$parent_ids = array_values( $featured );
$featured_product_ids = array_unique( array_merge( $product_ids, $parent_ids ) );
set_transient( 'wc_featured_products', $featured_product_ids );
return $featured_product_ids;
}
19 – Add custom field to edit address page
// add fields to edit address page
function woo_add_edit_address_fields( $fields ) {
$new_fields = array(
'date_of_birth' => array(
'label' => __( 'Date of birth', 'woocommerce' ),
'required' => false,
'class' => array( 'form-row' ),
),
);
$fields = array_merge( $fields, $new_fields );
return $fields;
}
add_filter( 'woocommerce_default_address_fields', 'woo_add_edit_address_fields' );
20 – Display onsale products catalog shortcode
function woocommerce_sale_products( $atts ) {
global $woocommerce_loop;
extract(shortcode_atts(array(
'per_page' => '12',
'columns' => '4',
'orderby' => 'date',
'order' => 'desc'
), $atts));
$woocommerce_loop['columns'] = $columns;
$args = array(
'post_type' => 'product',
'post_status' => 'publish',
'ignore_sticky_posts' => 1,
'posts_per_page' => $per_page,
'orderby' => $orderby,
'order' => $order,
'meta_query' => array(
array(
'key' => '_visibility',
'value' => array('catalog', 'visible'),
'compare' => 'IN'
),
array(
'key' => '_sale_price',
'value' => 0,
'compare' => '>',
'type' => 'NUMERIC'
)
)
);
query_posts($args);
ob_start();
woocommerce_get_template_part( 'loop', 'shop' );
wp_reset_query();
return ob_get_clean();
}
add_shortcode('sale_products', 'woocommerce_sale_products');
21 – Have onsale products
function woo_have_onsale_products() {
global $woocommerce;
// Get products on sale
$product_ids_on_sale = array_filter( woocommerce_get_product_ids_on_sale() );
if( !empty( $product_ids_on_sale ) ) {
return true;
} else {
return false;
}
}
// Example:
if( woo_have_onsale_products() ) {
echo 'have onsale products';
} else {
echo 'no onsale product';
}
22 – Set minimum order amount
add_action( 'woocommerce_checkout_process', 'wc_minimum_order_amount' );
function wc_minimum_order_amount() {
global $woocommerce;
$minimum = 50;
if ( $woocommerce->cart->get_cart_total(); < $minimum ) {
$woocommerce->add_error( sprintf( 'You must have an order with a minimum of %s to place your order.' , $minimum ) );
}
}
23 – Order by price, date or title on shop page
add_filter('woocommerce_default_catalog_orderby', 'custom_default_catalog_orderby');
function custom_default_catalog_orderby() {
return 'date'; // Can also use title and price
}
24 – Redirect add to cart button to checkout page
add_filter ('add_to_cart_redirect', 'redirect_to_checkout');
function redirect_to_checkout() {
global $woocommerce;
$checkout_url = $woocommerce->cart->get_checkout_url();
return $checkout_url;
}
25 – Add email recipient when order completed
function woo_extra_email_recipient($recipient, $object) {
$recipient = $recipient . ', your@email.com';
return $recipient;
}
add_filter( 'woocommerce_email_recipient_customer_completed_order', 'woo_extra_email_recipient', 10, 2);
And… that’s it! I hope you find will these snippets useful, they were all tested and they all work fine, but if you experience any trouble please let me know the comments section. Have fun! 😉
Very handy. I think code snippets worth more than a training book.
Thanks! 😉
Thanks for the snippets but how can i show the custom product variation field at the front-end? From 6 – ADD A CUSTOM FIELD TO A PRODUCT VARIATION
Hey, simply use get_post_meta() on the frontend: http://codex.wordpress.org/Function_Reference/get_post_meta
#22 is not working.
This should work:
// Set minimum order amount
add_action( 'woocommerce_checkout_process', 'wc_minimum_order_amount' );
function wc_minimum_order_amount() {
global $woocommerce;
$minimum = 50;
if ( $woocommerce->cart->total() add_error( sprintf( 'You must have an order with a minimum of %s to place your order.' , $minimum ) );
}
Code is stripped out 🙁
Thank you very much for these. Not only did post solve a problem i’ve been having, it’s given me a few more ideas for an upcoming project.
Thanks!
How can you make the product images bigger on the category pages? as well display more products too. (it currently displays only 10 products per page.)
Thanks
Define custom image size for products or use CSS code! 😉
Thanks for sharing the snippets! Real quick do you have any advice or resources on how to change the layout of the single product page? I’ve been trying to figure out the best practice for moving the entry-summary of a product into a sidebar and just keeping the title image and tabs in the main content area and everything else in a sidebar.
Thoughts? I’m sure someone has done this but haven’t come across an example yet.
– Thanks in advance
Use custom templates. Basically a custom template allows you to override WooCommerce default files and use your own custom files instead. Here is a quick tutorial that will explain you how to create your custom templates: http://docs.woothemes.com/document/template-structure/
Well, I do follow you recently. Really impressive to me. You do work as a Woocommerce just for 6 months? If so, I must confess that you do well. I am also work on online business but I do not have much experience. Love this post. The article is informative. Thanks for sharing. Great job!
Hey Tony, many thanks, i’m glad you liked my post, i guess i’ll write another one with new snippets very soon! 😉
Amazing post, very useful thank you Remi!
Do also have a snippet to place the add to cart button of a specific product anywhere of the page? Maybe also with variations?
Another interesting one would be to permanently show the add to cart button, even if you have variations.
yes i have such a snippet on my website, check it out
Great work mate. I was wondering if we could display “SOLD” under the product image on shop page of all sold items and new visitor could see all sold items. Is there anyway we can create markdown sale on all products in one go (best example would be markdown sale option in eBay stores).
I found following code
$link[‘label’] = apply_filters( ‘not_purchasable_text’, __( ‘Read More‘, ‘woocommerce’ ) );
which displays sold items on shop page. I want to change text from “Read More” to “SOLD”
I know, I can change it in woocommerce.css file but I would like to change it in child theme’s .css or functions.php file.
Could you please help to solve this issue.
I I Remi says:
I I November 12, 2013 at 4:26 am – Reply
I I Hey, simply use get_post_meta() on the frontend: I I http://codex.wordpress.org/Function_Reference/get_post_meta
thanks for your answer but i didnt know how to get the variable variation id, can you please write a example? my try was:
but i dont want a fix id, i want a variable post id. i hope you can help me, thanks.
Hi, Remi or Kamillo – did you find a solution for this – I’ve been searching everywhere to find a way to add the custom field to each variation, save it with variation data, and be able to retrieve it in my product detail page.
Would be great if anyone could help.
Thanks
Hi Remi,
just used the side-wide search but couldn’t find anything related to showing add to cart button with variations drop-down.
WooCommerce has a shortcode for showing just the add to cart button with price: [add_to_cart id=”99″] (http://docs.woothemes.com/document/woocommerce-shortcodes/#section-3)
Unfortunately, it doesn’t work with variations. Any ideas?
Hi, just came across your blog today but a few questions if anyone has some time. With #8, is there any way to make it work with Product Variations, so that if one variation is added to the Cart, the button says “Already in Cart” (as given in the code) but if you select the second product variation, it switches back to “Add to Cart”?
In other words, you have a Shirt with Red and Green options. If you add the Red Shirt to the cart, the button should change but when you go back and select the Green shirt, it should not say that the product is already in the Cart.
Second question, would you have any code snippets to allow the customer to select how many products they want shown on the Shop Page or even after a Search is returned. I know you can edit this manually to say 12 products but if the customer wanted to view 24, 36 or All Results on one page, is there any code that allows the user to select these options?
Last question, is it possible to disable the “click option of the product preview image” on a Single Product page at a “Product by Product” level or even a “Category” level. I realize you can disable the Lightbox but when you click the image, it still opens a tab with the image of the product. So I wanted to know if I can disable this “click” so that nothing registers but I still want to keep the click on for certain products. For example with Virtual Products, I might use a product placeholder and clicking on that product image is of no use to the customer. However, if I have a physical product then yes I would want the customer to click the Lightbox and see more pictures of the product.
Any help or pointers in the right direction would be appreciated.
I’d like to setup two different menus for my widget area on my woocommerce pages, for two different department categories? Any suggestions? can I place a line of code in functions.php to say on Category (blue) to show a different “template page” or widget area?
Hi, thanks a lot for this post, amazing! One quick question: Will these adjustments disappear if I update Woocommerce? Cheers
Nop!
Hi there!
I’m not a PHP guy, but I am a web designer with emphasis on the design aspect of it.
My question is on #6.
All I want is a field where the customer can enter personalized text for the product. I know that’s a premium add on through Woo but I hardly think it’s worth the $45. Especially for a site that is only selling one product. Will that do it?
Also, I copied the code and pasted it at the end of the functions.php file. I don’t see any difference. Where should that show up in the Admin area?
Sorry for my ignorance.
Thanks!
Very helpful. Thanks! I was able to change my add to cart button text.
Is there a snippet to add product colors under each product on the shop page?
Im using a plugin to make the colors show on the single product page but would love to have a mini version of those color boxes under each product on the shop page.
I am trying #5, but no luck there. I tried changing the array field with product category id but doesn’t work. Could you help, please?
It works when I am not using dropdown option. But i was wondering if it was possible with dropdown option.
Thanks
Tanvir
Thanks for all the info. You would not happen to have some code to remove the return to shop button from the empty cart.
Is there any way to make visible the cart to a particular product category and not visible in the other categories? Thank you
Hello, this is with respect to Product Add Ons. Would you know if it is possible to save the content of a form so that when the user leaves the page and comes back, they do not have to fill out the information again? So for example they come to the product page, choose “option A”, “option B” and “enter a message in the text box.”
They click on Add to Cart but then realize they have to edit their entry. So when I hit the back button, I realize that contents of the form are cleared and I have to make the selections again. How do I store the selections, so that the user can edit what they previously entered?
Any idea how to exclude a category from the “Top Rated Products” widget? I tried modifying some of your code (ie: exclude category from category widget), but couldn’t get it to work. Thanks!
Thank you for Number 13! The only thing is for me if you returned nothing, then no title was shown, so I just did an else statement:
add_filter( ‘woocommerce_page_title’, ‘woo_shop_page_title’);
function woo_shop_page_title( $page_title ) {
if($page_title == “Shop”) {
return “”;
}else{
return $page_title;
}
}
and everything worked perfectly. Much appreciated!
thanks! a few of them was very useful for me. One question, i use a shortcode for displaying recent products on my homepage, and i need to exclude one category. Do you have any pointers for achieving this? The shortcode i use is
Thanks!
Hi! I was looking for how to remove category for a long time, thanks for that, and small question, how to remove a few categories?
Thanks for the snippets… Do you know how to remove “add to cart” button and make it like “Call for Price”..and Also change “Free” in products that has a 0 price? Thank you!
Hi Remi!
In respect to #3 – REMOVE PRODUCT CATEGORIES FROM SHOP PAGE
…Is there a way rather than having ALL products listed on the SHOP base page, to tweak it to just a few catagories? E.g show 4 feature products, 4 newly added products and 4 best selling products on each line?
Thanks!
I tried number 23 – Order by date on shop page. Oddly enough it returned the sort by newness on the shop page when I was logged in and when I was logged out it went to sort by default but did not sort by date. Any thoughts on why I am achieving different results when logged in?
Are you using any caching plugins? If so, clear your cache.
Hi,
I want to this type look of my main page… as is it possible so pls share me… I want main page similar to qeemat.com
1st Latest Mobile Category
2nd Latest Laptop Category
3rd Latest Tablet Category
etc etc….
Yes, you can use the “products by category slug” WooCommerce Shortcode.
Hello,
I am looking for a method to hide/disable/ products with blank or zero price.
After an automatic import I sometimes have a bunch of products with no price that I’d like to not be shown unless another import sets the correct value.
Hi! great and useful post! thank you very much!
Could I make a question? I need to show a number with the products on sale, something like “15 products of 50 on sale”. Is there a snippet for this?
thank you very much!
This is actually on the post, have a look at snippet 21 – HAVE ONSALE PRODUCTS.
ups! so sorry! thank you very much!!!
Thank you & nice work !
For your first snippet I can’t adapt the code to coupon.
Do you have a trick ?
hi,
Woocommerce theme by default hides “Options values & Grand Total value” if option values are 0. Is it possible not to remove these divs, if value even 0?
Thanks
12 – LIST WOOCOMMERCE PRODUCT CATEGORIES
This code did not work for me. It actually broke my website 🙁
make sure you embed the code between PHP tags, that’s a common mistake when copying code
Hi Remi,
Do you have any tips to shorten (truncate) long products title in WooCommerce shop pages?
Regards,
Dhruba
Regarding #3: I’ve tried to accomplish this now for about 3 weeks. Trying so many different ways with no joy. I guess I’m not filling in what I’m supposed to correctly, but I’m not a coder and new to WP/WooCommerce. The only thing keeping me from going live is to remove just 1 category from showing on my shop page. 🙁 Can you please help me out?
Hi! I have used your code “8 – Display “product already in cart” instead of “add to cart” button” with woocommerce 2.0.20 and it was GREAT, worked just fine. I updated yesterday to woocommerce 2.1 and it is no longer working…
Do you know why this might be??
THANKS! 🙂
Looks like they changed the filter name so it has a prefix (as it should have been to start with) the new filter look like this:
I’ll update the post.
I vaguely understand how #6 works but I am not sure what file this goes in. Does it work in functions.php or somewhere else.
Yes you can add it in functions.php. Personally I prefer to make a new file called woocommerce-tweaks.php or something similar (then use require_once() in functions.php to load the file) where I add all my additions, that keeps my theme nice and clean 😉
For WC 2.1+
Remi,
I’m deploying my first woocommerce sites this month, and your snippet library is going to be very helpful. I’ve got a simple issue for which I’m sure you have a quick fix: First client is using the ‘Name Your Price’ plugin, which works well. Unfortunately, the ‘Add to Cart’ button exists not just on the PRODUCT page, but the product CATEGORY and SEARCH pages as well. Clicking an ‘Add to Cart’ button anywhere other than the product page adds the item to the cart at a value of $0. How do I display the ‘Add to Cart’ button ONLY on the Product page?
Hello,
This is a great list of snippets. Thank you! I am looking for something a bit different and wonder if you might share. What I would like to be able to do is to add categories to the shop page drop-down list. I’ve found functions for adding attributes or for sorting categories on the page itself, but I would like the user to be able to choose categories from the drop-down. Is this possible?
Thank you!
I’m not quite understanding what you want Wendy…the categories in the drop-down list are based on the categories you add in your WordPress dashboard. Have a look at the WooCommerce docs here – http://docs.woothemes.com/document/managing-product-taxonomies/
OK, so here’s a stupid question…but am I placing these snippets in my custom CSS editor, or in a php file somewhere?
They would go in your functions.php file.
Ahem…yes, I reread the top of the page and saw that. How bashful it made me feel. So sorry! Dare I ask, does it have to be a child theme, or do changes to a functions.php file survive them upgrades?
No worries Valerie, it’s more fun to jump into the code then read the post 😉 It’s always best to use a child theme. However, if this is your personal site lets say and you’ll never be changing the theme because you made it, then it’s fine to add it to the parent them. If you think one day you’ll switch themes or your aren’t the developer and might have to update in the future then use a child theme.
Do you know if there is a way to hide products after a specific date? We sell tickets to trips and have a cutoff date 3-4 days prior to the trip.
I’m not sure if there is any way of doing this. This is not really a WooCommerce snippet but more of a WordPress snippet (auto drafting/deleting posts). There is a plugin out there called “Post Expirator” I haven’t tested it myself, but might be worth checking out.
Hey Remi, cool post, I really liked it!
Just a question though, would you know if there is a way of changing the Price label to display “Out of Stock” when there is currently no stock available?
Cheers
Damien
Hi Remi,
Is there any code to show add to cart to product listing? E.g I have a product category selling routers, and when user click on this category, the list of routers appear and I would like to have an add to cart (as an option) instead of user having to go in to detail in order to add to cart.
Thanks
I want to get total order of a product by product id in this month, or date?
Can you help me
Awesome post really like it.. I am a WordPress Developer…
This is SO helpful – THANKS!
Thanks! 😉
I couldn’t get #3 (remove categories from product page) to work… did anyone else have a problem? I put it in my funtions.php and used the slug names to try and remove a few categories but they never left…
This is to remove products in certain categories from your main products archive (not singular product post). If you are aware of this and it’s not working for you, make sure you aren’t using the WooCommerce shortcode or your theme isn’t overriding the default archive. This will only work if you are on the products archive page and also if it’s defined as the shop in the WooCommerce settings.
ps: I noticed Remi also added “! is_user_logged_in()” so it won’t work if you are logged in either. I’m not sure why he added this, my guess is for debugging purposes.
AJ, thanks for the clarification. This isn’t what I was looking for. I recently took over managing a friend’s site for her. She originally had some 600+ products and probably 50 categories. She decided she only wanted to display her line of products (<20). I "hid" all of the other products by changing their visibility (just in case she changed her mind later and wanted to bring all of the other products back some day), but the categories don't have an option to hide them. I didn't want to delete them either because those 600+ products are still associated with all of these categories that I don't currently need, and again, should she change her mind, I didn't want to redo all of the work someone already did. So, basically, I was just trying to find a way to hide bunch of categories from the shop without deleting them. If you are anyone else knows a solution for me, i'd be grateful! Thanks again.
When you say hiding the categories from the shop though, do you mean under the product description where it says “Category:”? Or on the page that displays all the categories with a thumbnail? Or on the sidebar category widget? If you can specify, I can provide a solution 😉
Thanks AJ. If you look here susan-hopkins.com/lifestyle/ you will see the list of categories down the left side. The Blanco theme has an option to turn the categories sidebar on or off but I was hoping to keep it and hide all but a handful of the categories.
Looking at the source code this category widget isn’t a standard WP or WooCommerce widget. You are adding it via a plugin or the theme itself, you should try contacting the Blanco theme developer for assistance or the plugin developer if that widget is being added via a plugin.
ps: The easiest solution though is for you to just create a new custom menu for your categories at Appearance->Menu and then use the custom menu widget.
That’s a great idea! Thank you!!
Hello Remi and thank you for a great set of snippets! I have a question regarding the WooCommerce settings panels:
– is it possible, and how, to previously define a few of the settings and somehow hide them from the Shop Manager?
I need to have a few of these already setup by default for the Shop Manager (for instance, don´t activate taxes in the Taxes tab, prices already have tax, etc.) and hide them from him so that he cannot change them.
Any help is greatly appreciated. Thank you.
Hey Mentol, have a look at the WooCommerce Settings API page.