How to Link to the Current Category in WordPress
Displaying a link to the current post’s category in WordPress is easy to do and I will show you how you can do it via 3 different methods: using custom code, using the Total WordPress theme or via the Yoast SEO breadcrumbs.
Option 1: Using Custom Code
Simply paste this code wherever you want your category link to appear. This will display a link to the first category of your post. This code can be placed in any theme template file, even outside the loop, but it won’t work when placed in functions.php unless it’s hooked into an action hook that runs once WordPress has initialized such as the wp hook.
<?php
// Display the first category.
$first_cat = get_the_category()[0] ?? '';
if ( $first_cat ) {
echo '<a href="' . esc_url( get_category_link( $first_cat->cat_ID ) ) . '">' . esc_html( $first_cat->cat_name ) . '</a>';
}
?>
Linking to a custom Taxonomy
If you want to display the first category link for custom taxonomy then the code is a bit different since you can’t use the get_the_category()
function as that will only return categories you must instead use the get_the_terms()
function. Below is an example snippet showing how to display a link to the current post’s first term assigned to the “portfolio_category” taxonomy.
<?php
// Display the first portfolio_category.
$first_term = get_the_terms( get_post(), 'portfolio_category' )[0] ?? '';
if ( $first_term ) {
echo '<a href="' . esc_url( get_category_link( $first_term ) ) . '">' . esc_html( $first_term->name ) . '</a>';
}
?>
Creating a Helper Function
I find it’s always best to create a helper function for this sort of thing as you will probably want to use the same code multiple times in your project. For example you may want to display the first category link in your archive entries and also in your singular posts.
Here is a helper function you can use to display the first term link of a post. Notice I’m not saying “category” since it can be used for any taxonomy 😉
// Returns a link to the first term of a post for a given taxonomy.
function wpexplorer_get_first_term_link( string $taxonomy = 'category' ): string {
$term_link = '';
$first_term = get_the_terms( get_post(), $taxonomy )[0] ?? '';
if ( $first_term ) {
$term_link = '<a href="' . esc_url( get_category_link( $first_term ) ) . '">' . esc_html( $first_term->name ) . '</a>';
}
return $term_link;
}
And you can use the function like such:
<?php echo wpexplorer_get_first_term_link(); ?>
Adding Support for the Yoast Primary Category
The Yoast SEO plugin includes a built-in feature that allows you to set a specific category as the “Primary” category for a given post. I believe this functionality is used for breadcrumbs. In the snippets above the code will return the “first” category assigned to a post but if a post has multiple categories assigned to it, this may not be the category you want to display on your site.
By using the Yoast SEO primary category function you can specify what category to display on your the frontend of your site to your visitors. This is how you can “grab” the primary category:
if ( class_exists( 'WPSEO_Primary_Term' ) ) {
$taxonomy = 'category';
$yoast_primary_term_class = new WPSEO_Primary_Term( $taxonomy, get_the_ID() );
if ( $yoast_primary_term_class && is_callable( [ $yoast_primary_term_class, 'get_primary_term' ] ) ) {
$yoast_primary_term = $yoast_primary_term_class->get_primary_term();
if ( $yoast_primary_term && term_exists( $yoast_primary_term, $taxonomy ) ) {
$primary_term = $yoast_primary_term;
}
}
}
This code will simply return the primary term, so now lets update our helper function to include support for the Yoast primary term. The resulting code would look like this:
// Returns a link to the first term of a post for a given taxonomy.
function wpexplorer_get_first_term_link( string $taxonomy = 'category' ): string {
$term_link = '';
if ( \class_exists( '\WPSEO_Primary_Term' ) ) {
$yoast_primary_term_class = new \WPSEO_Primary_Term( $taxonomy, get_the_ID() );
if ( $yoast_primary_term_class && is_callable( [ $yoast_primary_term_class, 'get_primary_term' ] ) ) {
$yoast_primary_term = $yoast_primary_term_class->get_primary_term();
if ( $yoast_primary_term && term_exists( $yoast_primary_term, $taxonomy ) ) {
$first_term = get_term( $yoast_primary_term, $taxonomy );
}
}
}
$first_term = $first_term ?? get_the_terms( get_post(), $taxonomy )[0] ?? '';
if ( $first_term ) {
$term_link = '<a href="' . esc_url( get_category_link( $first_term ) ) . '">' . esc_html( $first_term->name ) . '</a>';
}
return $term_link;
}
Option 2: Using the Total WordPress Theme
If you are one of our Total theme customers (awesome) you may be working with a dynamic template and looking for a method to display links to the current post category in your template. Luckily this is very easy to do!
Simply insert the “Post Terms” element into your template and modify the settings to fit your needs. Below is a screenshot taken from the “Mason” demo using the WPBakery Page Builder in the backend view.
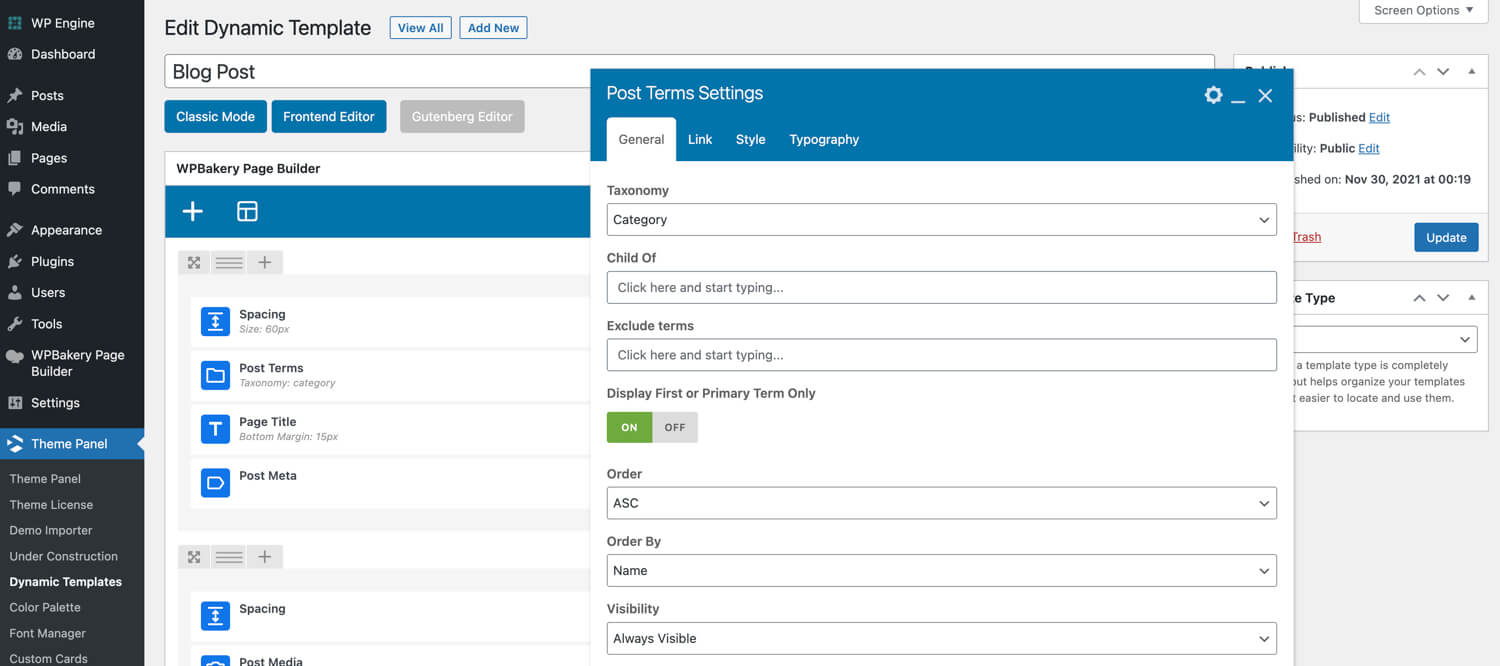
With Total you’ll have many options to style the way the category link works, you can choose to display all the categories or only the first/primary category and of course the element includes support for custom taxonomies.
This is what the element looks like on the live site for referrance:
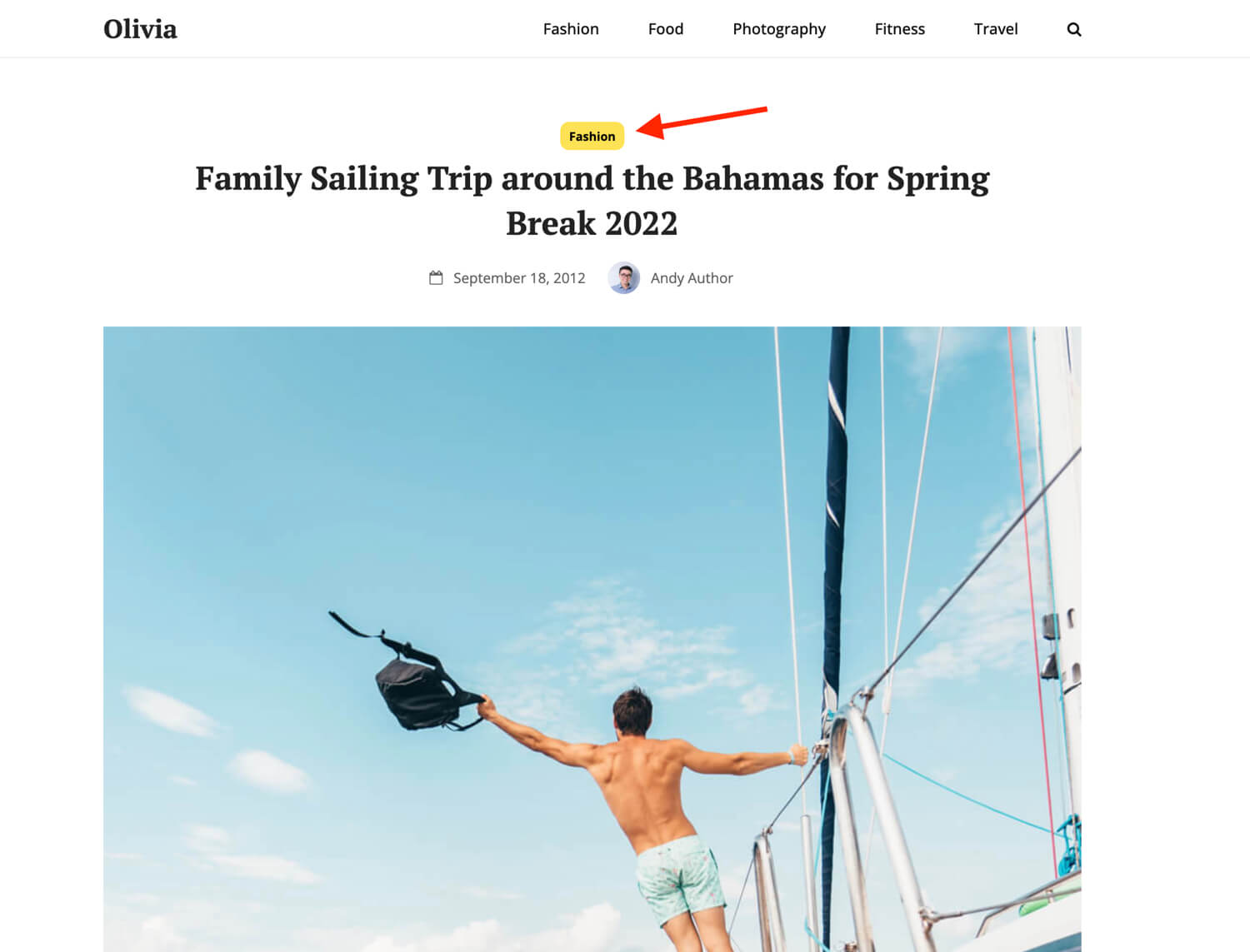
Option 3: Using Yoast SEO Breadcrumbs
Your other option is to simply use the breadcrumbs features built into the Yoast SEO plugin. Generally when displaying the current category for a post it’s a good idea to display it in your breadcrumbs because it provides an easy navigation across your site for users.
Breadcrumbs may also assist in your SEO efforts. Many free and premium WordPress themes actually use and recommend Yoast SEO for adding breadcrumbs because it is easy & effective.
Adding Yoast Breadcrumbs in Block Based Theme
If you are using a block based theme adding the Yoast breadcrumbs is very easy to do.
First go to Appearance > Editor click on Templates and then locate the Single template to open your single template design:
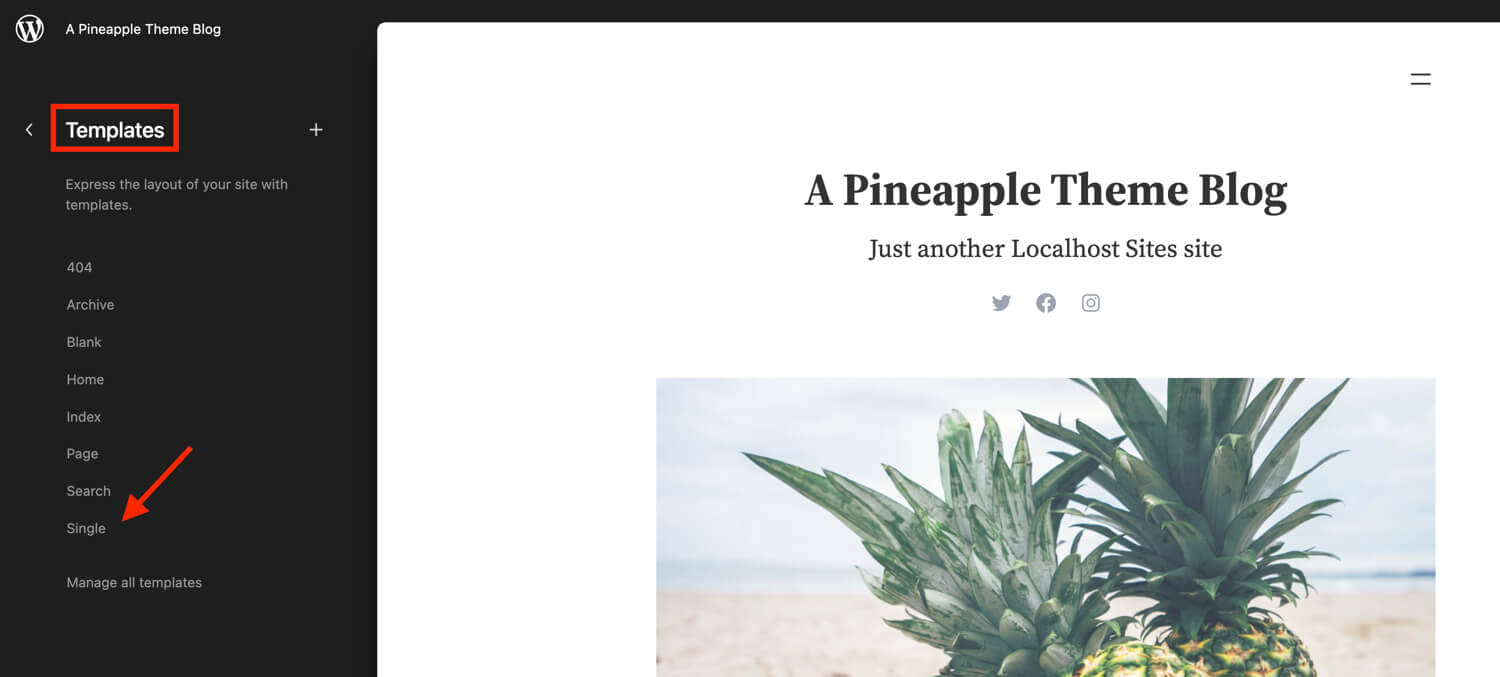
Now you will probably want to insert a new Group block and inside it insert the Yoast Breadcrumbs block:
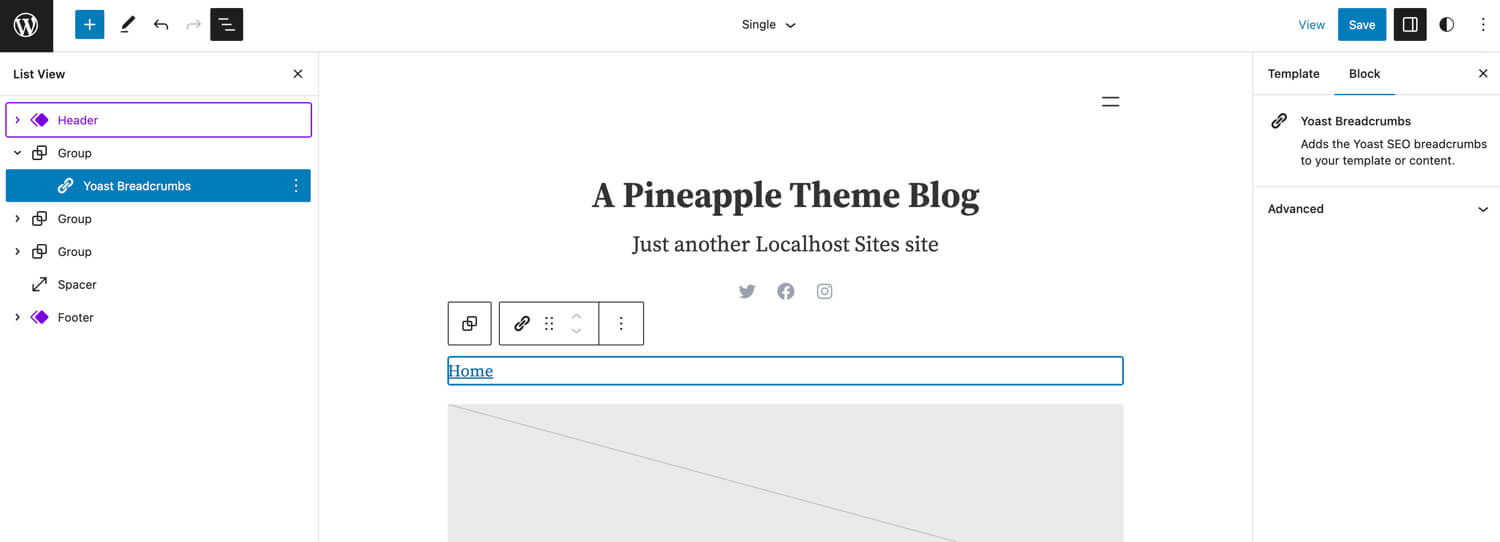
Since you are working in the side editor you will of course not see anything in the breadcrumbs besides the home link but rest assured on the frontend you will see the whole trail.
Additionally, we’ve only added the breadcrumbs to the single template, if your theme has multiple post types you will probably need to edit multiple templates and if you want the breadcrumbs added to archives as well as pages you will need to modify those templates as well.
Adding Yoast Breadcrumbs in your Classic Theme
If you are using a non block based theme (aka a classic theme) you’ll first need to make sure your theme is compatible with the Yoast SEO breadcrumbs. If it’s not this is easy to fix. Simply paste the following code into your theme file where you want to show your breadcrumbs (usually single.php or page.php above the page title):
if ( function_exists('yoast_breadcrumb') ) {
yoast_breadcrumb('<div id="breadcrumbs-trail">','</div>');
}
Note: Most premium themes already have built-in breadcrumbs. If you are using a premium theme and don’t see any breadcrumbs you should contact the theme developer for support. Only add this custom code if you are working with your own custom theme.
Setup Yoast SEO Breadcrumbs
Now that you have added the Yoast SEO block via your block based theme or added the custom code to your classic theme you will want to set up Yoast Breadcrumbs. For this you’ll want to go to the Yoast Settings panel, click on Advanced and then click on Breadcrumbs. Once you have the breadcrumbs panel open locate the Breadcrumbs for Post Types section where you can define the category or other taxonomy to display in your breadcrumbs trail.
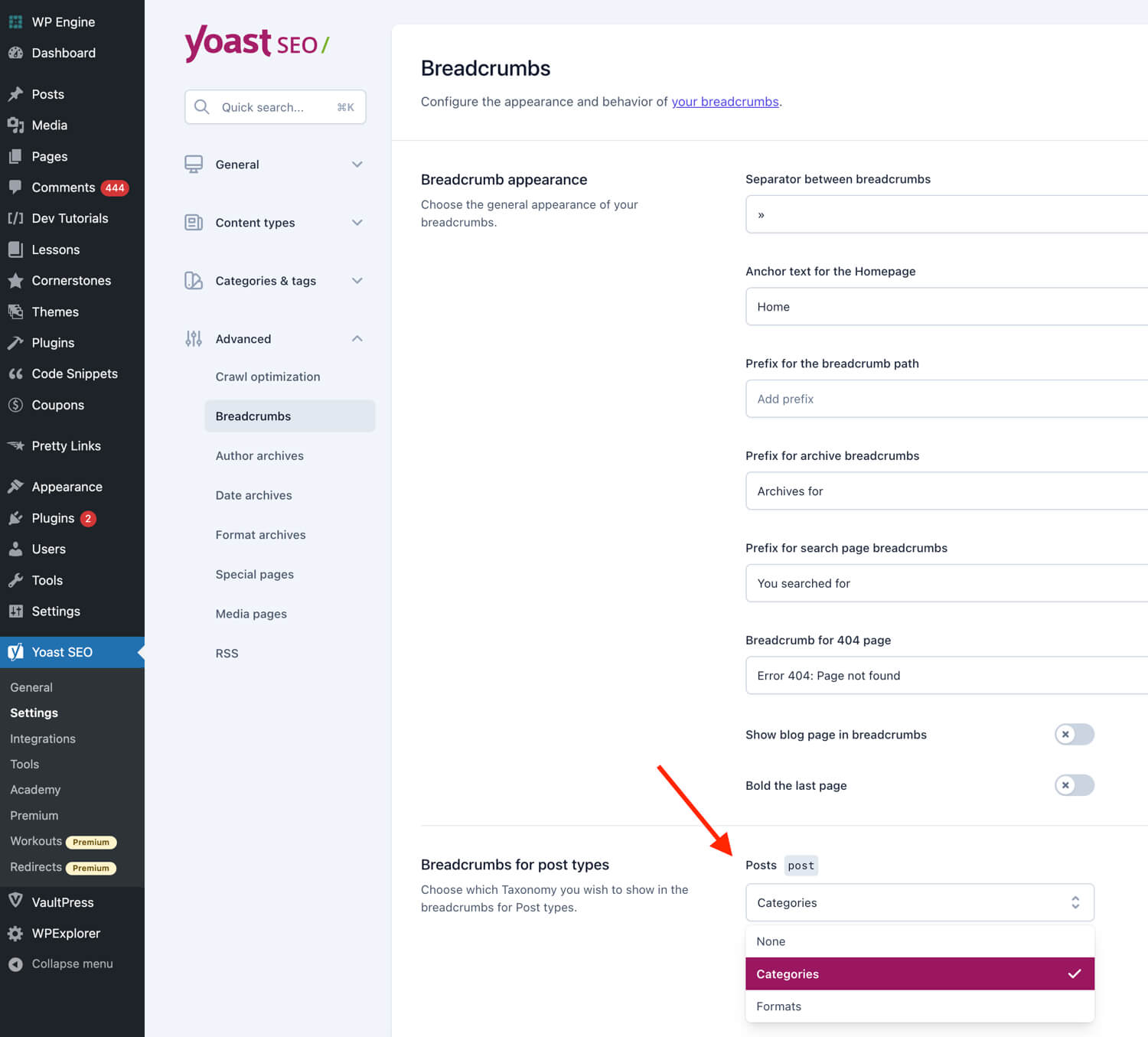
Displaying Category Links in WordPress Isn’t Hard
As you can see displaying the category link in WordPress isn’t hard at all and it can be done easily with a little custom code or you can simply display it in your breadcrumbs trail using a plugin like Yoast SEO.
I believe it’s important to display a link to the current post category because visitors on your site may want to look at related content and having a link to the category can help guide your visitors to other content making them happy but also increasing your pageviews so it’s also great for SEO.
Really thank you, must the code i find in the web dont work outside the Loop.
Thanks a lot.
Awesome, thanks man, was looking for this for ages.
Thank you!
Thank you, it helped me a lot!
Brilliant! Thanks, Wondered how do I now get a link for the root category (top-level parent) rather than the immediate parent category? Any ideas?
Hi Stuart,
Have a look at the get_category_parents function.
Thanks
Solved my problem! Thanks so much!
This helps me alot since my client’s web server couldn’t support breadcrumb functionality. So I had to be creative to link a single post to its category archive page. which also not actually the category page, as I had to populate the posts to other kind of archive page :D. The category link then redirected to that other kind of archive page.
Thank you! 🙂